JavaScript: Reverse the order of the characters in the string
JavaScript fundamental (ES6 Syntax): Exercise-153 with Solution
Reverse Characters in String
Write a JavaScript program to reverse the order of characters in the string.
- Use the spread operator (...) and Array.prototype.reverse() to reverse the order of the characters in the string.
- Combine characters to get a string using String.prototype.join('').
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'reverseString' that reverses the characters in a string
const reverseString = str =>
// Convert the string to an array of characters, reverse the array, and join the characters back into a string
[...str].reverse().join('');
// Test the 'reverseString' function with different input strings
console.log(reverseString('php')); // Output: 'php' (reversed)
console.log(reverseString('foobar')); // Output: 'raboof' (reversed)
Output:
php raboof
Visual Presentation:
Flowchart:
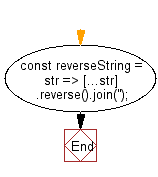
Live Demo:
See the Pen javascript-basic-exercise-153-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that reverses the characters in a string while preserving the positions of non-alphabet characters.
- Write a JavaScript function that reverses a string recursively without using built-in reverse methods.
- Write a JavaScript program that reverses only the letters in a string, leaving numbers and symbols in their original order.
- Write a JavaScript program that reverses the string and then interleaves it with the original string.
Go to:
PREV : Round Number to Digits.
NEXT : Object from Properties Failing Function.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.