JavaScript: Get an array of lines from the specified file
JavaScript fundamental (ES6 Syntax): Exercise-159 with Solution
Read File Lines into Array
Write a JavaScript program to get an array of lines from the specified file.
- Use fs.readFileSync() to create a Buffer from a file.
- Convert buffer to string using buf.toString(encoding) function.
- Use String.prototype.split(\n) to create an array of lines from the contents of the file.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Import the 'fs' module for file system operations in Node.js
const fs = require('fs');
// Define a function 'readFileLines' that reads the contents of a file and returns an array of lines
// It takes one parameter:
// 1. 'filename': The name of the file to read
const readFileLines = filename =>
// Read the contents of the file synchronously using 'fs.readFileSync'
fs
.readFileSync(filename)
// Convert the buffer to a UTF-8 encoded string and split it into an array of lines using '\n' as the delimiter
.toString('UTF8')
.split('\n');
// Call the 'readFileLines' function with the filename 'test.txt' and store the result in 'arr'
let arr = readFileLines('test.txt');
// Log the array 'arr' containing the lines read from the file
console.log(arr); // Logs ['line1', 'line2', 'line3']
Flowchart:
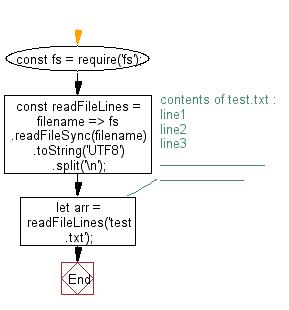
Live Demo:
See the Pen javascript-basic-exercise-159-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that reads a text file and splits its contents into an array of lines using FileReader API.
- Write a JavaScript function that accepts a file input and returns an array of non-empty lines from the file.
- Write a JavaScript program that reads a file asynchronously and processes its content into an array of trimmed strings.
- Write a JavaScript program that converts a file’s content into an array of lines, filtering out comment lines starting with "#".
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a function that invokes the provided function with its arguments arranged according to the specified indexes.
Next: Write a JavaScript program to mutate the original array to filter out the values specified, based on a given iterator function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.