JavaScript: Return 1 if the array is sorted in ascending order, -1 if it is sorted in descending order or 0 if it is not sorted
JavaScript fundamental (ES6 Syntax): Exercise-188 with Solution
Array Sort Order
Write a JavaScript program that returns 1 if the array is sorted in ascending order. It returns -1 if it is sorted in descending order or 0 if it is not sorted.
- Calculate the ordering direction for the first pair of adjacent array elements.
- Return 0 if the given array is empty, only has one element or the direction changes for any pair of adjacent array elements.
- Use Math.sign() to covert the final value of direction to -1 (descending order) or 1 (ascending order).
Sample Solution:
JavaScript Code:
// Define a function 'isSorted' that checks if the array 'arr' is sorted in either ascending or descending order
const isSorted = arr => {
// Determine the initial direction of sorting by comparing the first two elements
let direction = -(arr[0] - arr[1]);
// Iterate over each element in the array
for (let [i, val] of arr.entries()) {
// If the direction is not set (0), calculate the direction based on the previous elements
direction = !direction ? -(arr[i - 1] - arr[i]) : direction;
// If it's the last element in the array, return 0 if the array is sorted, otherwise return the direction
if (i === arr.length - 1) return !direction ? 0 : direction;
// If the current element and the next one violate the sorting order, return 0
else if ((val - arr[i + 1]) * direction > 0) return 0;
}
};
// Test cases to check if arrays are sorted
console.log(isSorted([0, 1, 2, 2])); // 1 (ascending order)
console.log(isSorted([4, 3, 2])); // -1 (descending order)
console.log(isSorted([4, 3, 5])); // 0 (not sorted)
Output:
1 -1 0
Visual Presentation:
Flowchart:
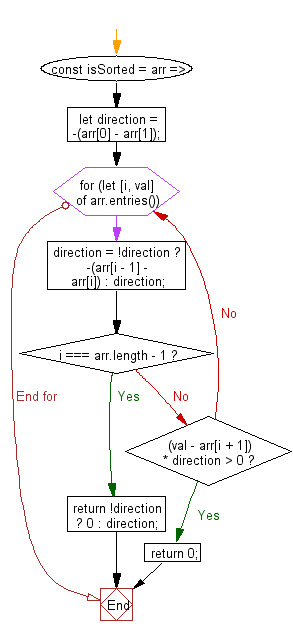
Live Demo:
See the Pen javascript-basic-exercise-188-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that determines if an array is sorted in ascending order, descending order, or not sorted at all.
- Write a JavaScript function that iterates through an array and returns 1 if sorted ascending, -1 if descending, and 0 otherwise.
- Write a JavaScript program that compares adjacent elements to decide the overall sort order of the array.
- Write a JavaScript function that uses Array.every() to verify if an array is monotonically increasing or decreasing.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check if the given argument is a string.
Next: Write a JavaScript program that will return true if an object looks like a Promise, false otherwise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.