JavaScript: Check whether a string is lower case or not
JavaScript fundamental (ES6 Syntax): Exercise-197 with Solution
Write a JavaScript program to check whether a string is lower case or not.
- Convert the given string to lower case, using String.prototype.toLowerCase() and compare it to the original.
Sample Solution:
JavaScript Code:
// Define a function 'isLowerCase' that checks if the given string 'str' contains only lowercase letters
const isLowerCase = str =>
// Check if the given string 'str' is equal to its lowercase version
str === str.toLowerCase();
// Test cases to check if the strings contain only lowercase letters
console.log(isLowerCase('abc')); // true (all letters are lowercase)
console.log(isLowerCase('a3@$')); // true (all letters are lowercase)
console.log(isLowerCase('Ab4')); // false (one letter is uppercase)
Output:
true true false
Visual Presentation:
Flowchart:
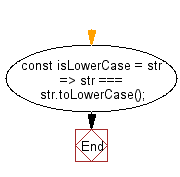
Live Demo:
See the Pen javascript-basic-exercise-197-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return true if the specified value is null, false otherwise.
Next: Write a JavaScript program to check if the given argument is a function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics