JavaScript: Return true if the given number is even, false otherwise
JavaScript fundamental (ES6 Syntax): Exercise-199 with Solution
Check If Even Number
Write a JavaScript program that returns true if the given number is even, false otherwise.
- Checks whether a number is odd or even using the modulo (%) operator.
- Returns true if the number is even, false if the number is odd.
Sample Solution:
JavaScript Code:
// Define a function 'isEven' that checks if the given number 'num' is even
const isEven = num =>
// Check if the remainder of 'num' divided by 2 is equal to 0
num % 2 === 0;
// Test cases to check if the numbers are even
console.log(isEven(3)); // false (the number is odd)
console.log(isEven(32)); // true (the number is even)
console.log(isEven(1)); // false (the number is odd)
console.log(isEven(0)); // true (zero is considered an even number)
Output:
false true false true
Visual Presentation:
Flowchart:
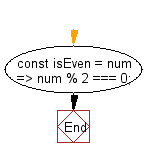
Live Demo:
See the Pen javascript-basic-exercise-199-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if a given number is even by using the modulo operator.
- Write a JavaScript function that tests a number for evenness and handles non-numeric input gracefully.
- Write a JavaScript program that determines even numbers in an array and returns a filtered array of even values.
- Write a JavaScript function that checks if a number is even by converting it to an integer and applying modulus 2.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check if the given argument is a function.
Next: Write a JavaScript program that will return true if a value is an empty object, collection, map or set, has no enumerable properties or is any type that is not considered a collection.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.