JavaScript: Extend a 3-digit color code to a 6-digit color code
JavaScript fundamental (ES6 Syntax): Exercise-20 with Solution
Expand 3-Digit to 6-Digit Color Code
Write a JavaScript program to extend a 3-digit color code to a 6-digit color code.
- Use Array.prototype.map(), String.prototype.split() and Array.prototype.join() to join the mapped array for converting a 3-digit RGB notated hexadecimal color-code to the 6-digit form.
- Array.prototype.slice() is used to remove # from string start since it's added once.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `extend_Hex` that extends a short hex color code to its full format.
const extend_Hex = shortHex =>
'#' +
shortHex
.slice(shortHex.startsWith('#') ? 1 : 0) // Remove '#' if present
.split('') // Split the string into an array of characters
.map(x => x + x) // Duplicate each character in the array
.join(''); // Join the array back into a string
// Example usage
console.log(extend_Hex('#03f')); // #0033ff
console.log(extend_Hex('05a')); // #0055aa
Output:
#0033ff #0055aa
Visual Presentation:
Flowchart:
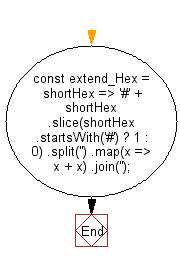
Live Demo:
See the Pen javascript-basic-exercise-1-20 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a 3-digit hexadecimal color code to its equivalent 6-digit format.
- Write a JavaScript function that checks if a color code is in 3-digit format and expands it by duplicating each digit.
- Write a JavaScript program that validates a 3-digit hex color and returns the expanded 6-digit version.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to remove specified elements from the right of a given array of elements.
Next: Write a JavaScript program to get every nth element in an given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.