JavaScript: Check if a given string is an anagram of another string (case-insensitive, ignores spaces, punctuation and special characters)
JavaScript fundamental (ES6 Syntax): Exercise-206 with Solution
Check Anagram
Write a JavaScript program to check if a given string is an anagram of another string (case-insensitive, ignores spaces, punctuation and special characters).
- Use String.prototype.toLowerCase() and String.prototype.replace() with an appropriate regular expression to remove unnecessary characters.
- Use String.prototype.split(''), Array.prototype.sort() and Array.prototype.join('') on both strings to normalize them, then check if their normalized forms are equal.
Sample Solution:
JavaScript Code:
// Define a function 'isAnagram' that checks if two input strings are anagrams of each other
const isAnagram = (str1, str2) => {
// Define a helper function 'normalize' to normalize the input string
const normalize = str =>
str
.toLowerCase() // Convert the string to lowercase
.replace(/[^a-z0-9]/gi, '') // Remove non-alphanumeric characters
.split('') // Convert the string to an array of characters
.sort() // Sort the characters in alphabetical order
.join(''); // Convert the array back to a string
// Check if the normalized form of the first string is equal to the normalized form of the second string
return normalize(str1) === normalize(str2);
};
// Test cases to check if the input strings are anagrams
console.log(isAnagram('iceman', 'cinema')); // true (both strings contain the same letters)
console.log(isAnagram('madam', 'madam')); // true (both strings are the same)
Output:
true true
Visual Presentation:
Flowchart:
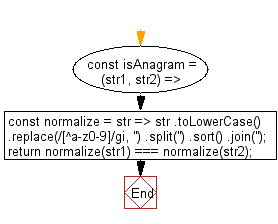
Live Demo:
See the Pen javascript-basic-exercise-206-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if two strings are anagrams by comparing sorted versions of the normalized strings.
- Write a JavaScript function that removes spaces and punctuation, then compares character frequencies to determine anagrams.
- Write a JavaScript program that uses a hash map to count characters in two strings and compares the counts.
- Write a JavaScript function that returns true if two strings are anagrams, ignoring case and special characters.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check whether the provided argument is array-like (i.e. is iterable).
Next: Write a JavaScript program to that will return true if the given string is an absolute URL, false otherwise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.