JavaScript: Get the last key that satisfies the provided testing function, otherwise undefined is returned
JavaScript fundamental (ES6 Syntax): Exercise-235 with Solution
Last Key Satisfying Condition
Write a JavaScript program to get the last key that satisfies the provided testing function, otherwise undefined is returned.
- Use Object.keys(obj) to get all the properties of the object.
- Use Array.prototype.reverse() to reverse the order and Array.prototype.find() to test the provided function for each key-value pair.
- The callback receives three arguments - the value, the key and the object.
Sample Solution:
JavaScript Code:
// Define a function 'findLastKey' to find the last key in an object that satisfies a provided testing function
const findLastKey = (obj, fn) =>
Object.keys(obj) // Get the keys of the object
.reverse() // Reverse the array of keys to search from end to start
.find(key => fn(obj[key], key, obj)); // Find the last key that satisfies the condition
// Test the 'findLastKey' function with a sample object and testing function
console.log(findLastKey(
{
barney: { age: 36, active: true },
fred: { age: 40, active: false },
pebbles: { age: 1, active: true }
},
o => o['active'] // Check if the 'active' property of the object is true
)); // Output: 'pebbles'
Output:
pebbles
Flowchart:
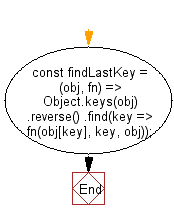
Live Demo:
See the Pen javascript-basic-exercise-235-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns the last key in an object for which a provided test function returns true.
- Write a JavaScript function that iterates over an object’s keys in reverse and returns the first key that satisfies a condition.
- Write a JavaScript program that searches an object for keys matching a predicate and returns the last matching key.
- Write a JavaScript function that uses Object.keys(), reverses the key array, and finds the last key meeting a criterion.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to flatten a given array up to the specified depth.
Next: Write a JavaScript program to get the first key that satisfies the provided testing function. Otherwise return undefined.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.