JavaScript: Create an element from a string
JavaScript fundamental (ES6 Syntax): Exercise-250 with Solution
Create Element from String
Write a JavaScript program to create an element from a string (without appending it to the document).
If the given string contains multiple elements, only the first one will be returned.
- Use Document.createElement() to create a new element.
- Use Element.innerHTML to set its inner HTML to the string supplied as the argument.
- Use ParentNode.firstElementChild to return the element version of the string.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'createElement' that creates a DOM element from the provided HTML string
const createElement = str => {
// Create a new div element
const el = document.createElement('div');
// Set the innerHTML of the div to the provided HTML string
el.innerHTML = str;
// Return the first element child of the div
return el.firstElementChild;
};
// Call 'createElement' with a sample HTML string and assign the result to 'el'
const el = createElement(
`<div class="container">
<p>Hello!</p>
</div>`
);
// Log the className property of the created element 'el'
console.log(el.className); // 'container'
Output:
container
Flowchart:
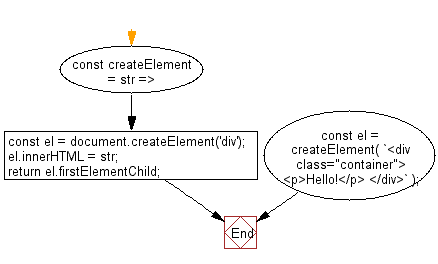
Live Demo:
See the Pen javascript-basic-exercise-250-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the current URL.
Next: Write a JavaScript program to write a JSON object to a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-250.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics