JavaScript: Check if two given numbers are approximately equal to each other
JavaScript fundamental (ES6 Syntax): Exercise-256 with Solution
Approximate Equality Check
Write a JavaScript program to check if two given numbers are approximately equal to each other.
- Use Math.abs() to compare the absolute difference of the two values to epsilon.
- Omit the third argument, epsilon, to use a default value of 0.001.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'approximatelyEqual' to check if two values are approximately equal within a specified epsilon range
const approximatelyEqual = (v1, v2, epsilon = 0.001) => Math.abs(v1 - v2) < epsilon;
// Test the 'approximatelyEqual' function with two values and a default epsilon value
console.log(approximatelyEqual(Math.PI / 2.0, 1.5708)); // true (approximately equal within the default epsilon range)
Output:
true
Flowchart:
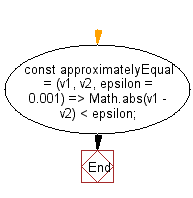
Live Demo:
See the Pen javascript-basic-exercise-157-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if two floating-point numbers are approximately equal within a given epsilon.
- Write a JavaScript function that compares two numbers and returns true if their difference is less than a small threshold.
- Write a JavaScript program that validates numerical approximations by comparing absolute differences against a tolerance.
- Write a JavaScript function that tests for near-equality using relative and absolute error margins.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return true if the provided predicate function returns true for at least one element in a collection, false otherwise.
Next: Write a JavaScript program to convert a 2D array to a comma-separated values (CSV) string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.