JavaScript: Converts a comma-separated values string to a 2D array
JavaScript fundamental (ES6 Syntax): Exercise-3 with Solution
CSV String to 2D Array
Write a JavaScript program to convert a comma-separated value (CSV) string to a 2D array.
Note: Use String.split('\n') to create a string for each row, then String.split(delimiter) to separate the values in each row. Omit the second argument, delimiter, to use a default delimiter of ,. Omit the third argument, omitFirstRow, to include the first row (title row) of the CSV string.
- Use Array.prototype.slice() and Array.prototype.indexOf('\n') to remove the first row (title row) if omitFirstRow is true.
- Use String.prototype.split('\n') to create a string for each row, then String.prototype.split(delimiter) to separate the values in each row.
- Omit the second argument, delimiter, to use a default delimiter of ,.
- Omit the third argument, omitFirstRow, to include the first row (title row) of the CSV string.
Sample Solution:
JavaScript Code:
// Source: https://bit.ly/2neWfJ2
// Define a function called `csv_to_array` that converts CSV data to an array.
const csv_to_array = (data, delimiter = ',', omitFirstRow = false) =>
// Split the CSV data by newline characters, map each row to an array of values split by the delimiter.
data
.slice(omitFirstRow ? data.indexOf('\n') + 1 : 0)
.split('\n')
.map(v => v.split(delimiter));
// Test cases
console.log(csv_to_array('a,b\nc,d')); // [['a', 'b'], ['c', 'd']]
console.log(csv_to_array('a;b\nc;d', ';')); // [['a', 'b'], ['c', 'd']]
console.log(csv_to_array('head1,head2\na,b\nc,d', ',', true)); // [['a', 'b'], ['c', 'd']]
Output:
[["a","b"],["c","d"]] [["a","b"],["c","d"]] [["a","b"],["c","d"]]
Flowchart:
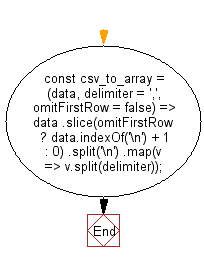
Live Demo:
See the Pen javascript-basic-exercise-1-3 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a CSV string into a two-dimensional array, correctly handling quoted commas.
- Write a JavaScript function that parses a CSV string with newline delimiters and returns an array of arrays.
- Write a JavaScript program that splits a CSV string into rows and columns while ignoring empty cells.
Go to:
PREV : Copy String to Clipboard.
NEXT : CSV to 2D Array of Objects.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.