JavaScript: Converts a specified number to an array of digits
JavaScript fundamental (ES6 Syntax): Exercise-7 with Solution
Number to Array of Digits
Write a JavaScript program to convert a specified number into an array of digits.
Note: Convert the number to a string, using the spread operator (...) to build an array.
- Use Math.abs() to strip the number's sign.
- Convert the number to a string, using the spread operator (...) to build an array.
- Use Array.prototype.map() and parseInt() to transform each value to an integer.
Sample Solution:
JavaScript Code:
// Convert a number into an array of its digits.
// Define a function called `digitize` that takes a number `n`.
const digitize = n =>
// Convert the number to a string, then split it into an array of characters.
[...`${n}`]
// Map each character to its corresponding integer value.
.map(i => parseInt(i));
// Test cases
console.log(digitize(123)); // Output: [1, 2, 3]
console.log(digitize(1230)); // Output: [1, 2, 3, 0]
Output:
[1,2,3] [1,2,3,0]
Visual Presentation:
Flowchart:
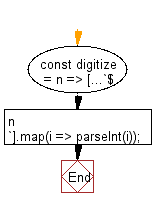
Live Demo:
See the Pen javascript-basic-exercise-1-7 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a given number into an array of its individual digits.
- Write a JavaScript function that accepts a number and returns an array of digits in reverse order.
- Write a JavaScript program that transforms a negative number into an array of digits, preserving the sign separately.
Go to:
PREV : Target Value in Nested JSON.
NEXT : Filter Specified Values from Array.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.