JavaScript: Invert the key-value pairs of an object, without mutating it
JavaScript fundamental (ES6 Syntax): Exercise-78 with Solution
Write a JavaScript program to invert the key-value pairs of an object, without mutating it. The corresponding inverted value of each inverted key is an array of keys responsible for generating the inverted value. If a function is supplied, it is applied to each inverted key.
- Use Object.keys() and Array.prototype.reduce() to invert the key-value pairs of an object and apply the function provided (if any).
- Omit the second argument, fn, to get the inverted keys without applying a function to them.
- The corresponding inverted value of each inverted key is an array of keys responsible for generating the inverted value. If a function is supplied, it is applied to each inverted key.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'invertKeyValues' to invert the keys and values of an object, optionally applying a function to the values.
const invertKeyValues = (obj, fn) =>
// Use Object.keys() to get an array of keys of the object, then reduce it to create a new object with inverted keys and values.
Object.keys(obj).reduce((acc, key) => {
// Apply the optional function to the value or use the value directly.
const val = fn ? fn(obj[key]) : obj[key];
// If the inverted value does not exist in the accumulator object, initialize it as an empty array.
acc[val] = acc[val] || [];
// Push the original key into the array associated with the inverted value.
acc[val].push(key);
return acc; // Return the updated accumulator object.
}, {});
// Example usage:
console.log(invertKeyValues({ a: 1, b: 2, c: 1 })); // Outputs: { '1': ['a', 'c'], '2': ['b'] }
console.log(invertKeyValues({ a: 1, b: 2, c: 1 }, value => 'group' + value)); // Outputs: { group1: ['a', 'c'], group2: ['b'] }
Output:
{"1":["a","c"],"2":["b"]} {"group1":["a","c"],"group2":["b"]}
Flowchart:
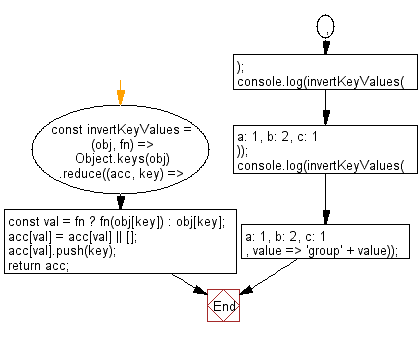
Live Demo:
See the Pen javascript-basic-exercise-78-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to iterate over all own properties of an object, running a callback for each one.
Next: Write a JavaScript program to take any number of iterable objects or objects with a length property and returns the longest one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics