JavaScript : Create an object composed of the properties the given function returns truthy for
JavaScript fundamental (ES6 Syntax): Exercise-97 with Solution
Object from Truthy Function Keys
Write a JavaScript program to create an object composed of the properties the given function returns truthy for. The function is invoked with two arguments: (value, key).
- Use Object.keys(obj) and Array.prototype.filter()to remove the keys for which fn returns a falsy value.
- Use Array.prototype.reduce() to convert the filtered keys back to an object with the corresponding key-value pairs.
- The callback function is invoked with two arguments: (value, key).
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'pickBy' function to pick key-value pairs from an object based on a filtering function.
const pickBy = (obj, fn) =>
// Filter the keys of the object based on the filtering function 'fn', then reduce them to build a new object.
Object.keys(obj)
.filter(k => fn(obj[k], k)) // Filter keys based on the filtering function.
.reduce((acc, key) => ((acc[key] = obj[key]), acc), {}); // Build a new object containing the filtered key-value pairs.
// Test the 'pickBy' function with an object and a filtering function.
console.log(pickBy({ a: 1, b: '2', c: 3 }, x => typeof x === 'number')); // Output: { a: 1, c: 3 }
Output:
{"a":1,"c":3}
Flowchart:
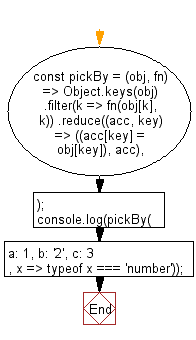
Live Demo:
See the Pen javascript-basic-exercise-97-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates an object containing only the key-value pairs for which a provided function returns truthy for the key.
- Write a JavaScript function that filters an object’s properties based on a callback applied to each key and returns the filtered object.
- Write a JavaScript program that iterates over an object and constructs a new object with properties passing a truthy test.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to pick the key-value pairs corresponding to the given keys from an object.
Next: Write a JavaScript program to filter an array of objects based on a condition while also filtering out unspecified keys.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.