JavaScript: Print the elements of an array
JavaScript Array: Exercise-10 with Solution
Write a JavaScript program that prints the elements of the following array.
Note : Use nested for loops.
Sample array : var a = [[1, 2, 1, 24], [8, 11, 9, 4], [7, 0, 7, 27], [7, 4, 28, 14], [3, 10, 26, 7]];
Sample Output :
"row 0"
" 1"
" 2"
" 1"
" 24"
"row 1"
------
------
Visual Presentation:
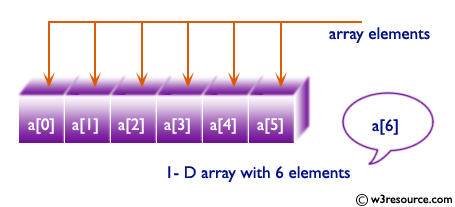
Sample Solution:
JavaScript Code:
// Declare and initialize a sample 2-D array
var a = [[1, 2, 1, 24], [8, 11, 9, 4], [7, 0, 7, 27], [7, 4, 28, 14], [3, 10, 26, 7]];
// Iterate through each row in the 2-D array
for (var i in a) {
// Output the current row index
console.log("row " + i);
// Iterate through each element in the current row
for (var j in a[i]) {
// Output the current element value
console.log(" " + a[i][j]);
}
}
Output:
row 0 1 2 1 24 row 1 8 11 9 4 row 2 7 0 7 27 row 3 7 4 28 14 row 4 3 10 26 7
Flowchart:
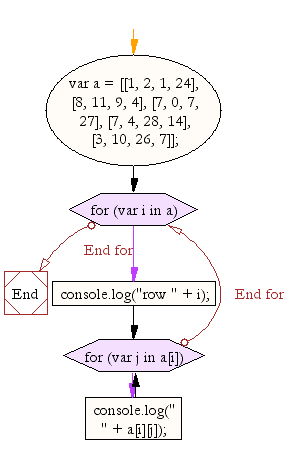
ES6 Version:
// Declare and initialize a sample 2-D array
const a = [
[1, 2, 1, 24],
[8, 11, 9, 4],
[7, 0, 7, 27],
[7, 4, 28, 14],
[3, 10, 26, 7]
];
// Iterate through each row in the 2-D array
for (const i in a) {
// Output the current row index
console.log(`row ${i}`);
// Iterate through each element in the current row
for (const j in a[i]) {
// Output the current element value
console.log(` ${a[i][j]}`);
}
}
Live Demo:
See the Pen JavaScript - Print the elements of an array- array-ex- 10 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program which accept a string as input and swap the case of each character.
Next: Write a JavaScript program to find the sum of squares of a numeric vector.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics