JavaScript: Compute the union of two arrays
JavaScript Array: Exercise-22 with Solution
Write a JavaScript program to compute the union of two arrays.
Sample Data:
console.log(union([1, 2, 3], [100, 2, 1, 10]));
[1, 2, 3, 10, 100]
Visual Presentation:
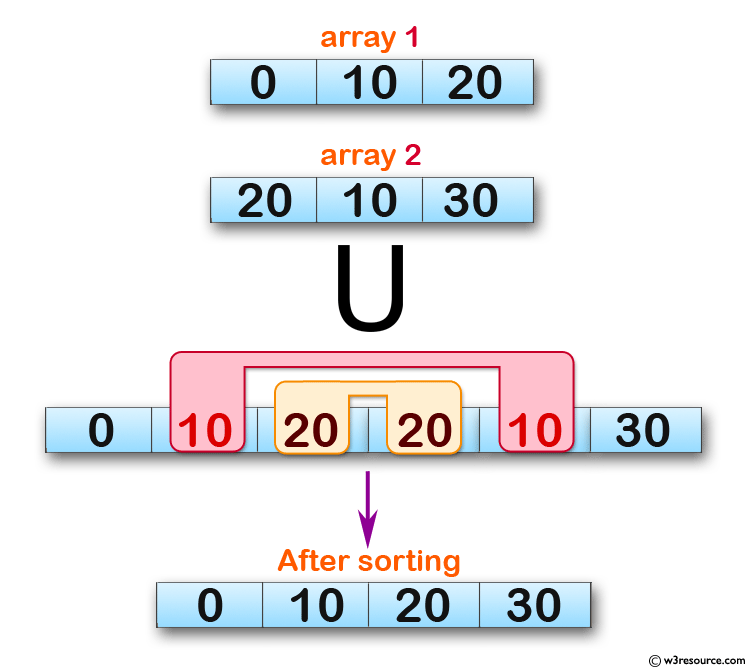
Sample Solution:
JavaScript Code:
// Function to find the union of two arrays
function union(arra1, arra2) {
// Check if either of the arrays is null, return undefined if true
if ((arra1 == null) || (arra2 == null))
return void 0;
// Initialize an empty object to store unique elements from both arrays
var obj = {};
// Iterate through the elements of arra1 in reverse order
for (var i = arra1.length - 1; i >= 0; --i)
// Use each element as a key in the object to store unique values
obj[arra1[i]] = arra1[i];
// Iterate through the elements of arra2 in reverse order
for (var i = arra2.length - 1; i >= 0; --i)
// Use each element as a key in the object to store unique values
obj[arra2[i]] = arra2[i];
// Initialize an empty array to store the result (union)
var res = [];
// Iterate through the properties of the object
for (var n in obj) {
// Check if the property belongs to the object (not inherited)
if (obj.hasOwnProperty(n))
// Push the unique value to the result array
res.push(obj[n]);
}
// Return the result array containing the union of the two input arrays
return res;
}
// Output the result of the union function with sample arrays
console.log(union([1, 2, 3], [100, 2, 1, 10]));
Output:
[1,2,3,10,100]
Flowchart:
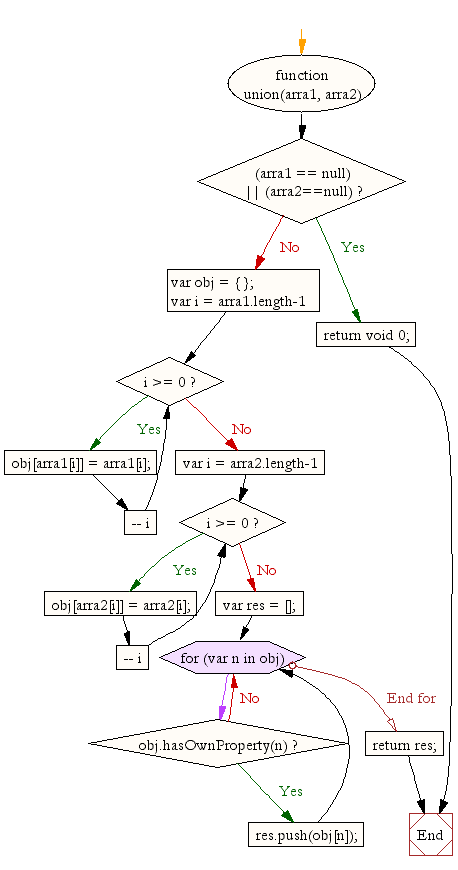
ES6 Version:
// Function to find the union of two arrays
const union = (arra1, arra2) => {
// Check if either of the arrays is null, return undefined if true
if ((arra1 == null) || (arra2 == null))
return void 0;
// Initialize an empty object to store unique elements from both arrays
const obj = {};
// Iterate through the elements of arra1 in reverse order
for (let i = arra1.length - 1; i >= 0; --i)
// Use each element as a key in the object to store unique values
obj[arra1[i]] = arra1[i];
// Iterate through the elements of arra2 in reverse order
for (let i = arra2.length - 1; i >= 0; --i)
// Use each element as a key in the object to store unique values
obj[arra2[i]] = arra2[i];
// Initialize an empty array to store the result (union)
const res = [];
// Iterate through the properties of the object
for (let n in obj) {
// Check if the property belongs to the object (not inherited)
if (obj.hasOwnProperty(n))
// Push the unique value to the result array
res.push(obj[n]);
}
// Return the result array containing the union of the two input arrays
return res;
};
// Output the result of the union function with sample arrays
console.log(union([1, 2, 3], [100, 2, 1, 10]));
Live Demo:
See the Pen JavaScript - Compute the union of two arrays - array-ex- 22 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to flatten a nested (any depth) array.
Next: Write a JavaScript function to find the difference of two arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics