JavaScript: Find if an array contains a specific element
JavaScript Array: Exercise-32 with Solution
Find Element in Array
Write a JavaScript function to find an array containing a specific element.
Test data:
arr = [2, 5, 9, 6];
console.log(contains(arr, 5));
[True]
Visual Presentation:
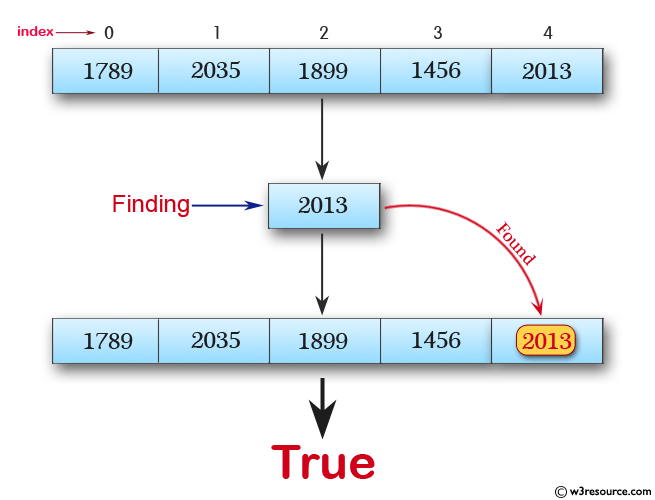
Sample Solution:
JavaScript Code:
// Function to check if an array contains a specific element
function contains(arr, element) {
// Iterate through the array
for (var i = 0; i < arr.length; i++) {
// Check if the current element is equal to the target element
if (arr[i] === element) {
// Return true if the element is found in the array
return true;
}
}
// Return false if the element is not found in the array
return false;
}
// Sample array
arr = [2, 5, 9, 6];
// Output the result of checking if the array contains the element '5'
console.log(contains(arr, 5));
Output:
true
Flowchart:
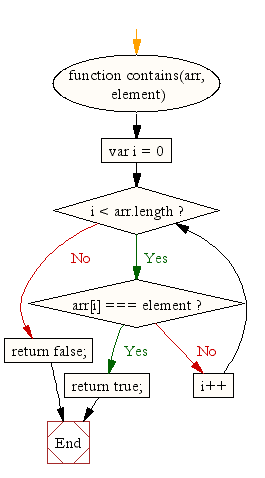
ES6 Version:
// Function to check if an array contains a specific element
const contains = (arr, element) => {
// Iterate through the array using the Array.prototype.some method
return arr.some(item => item === element);
};
// Sample array
const arr = [2, 5, 9, 6];
// Output the result of checking if the array contains the element '5'
console.log(contains(arr, 5));
Live Demo:
See the Pen JavaScript - Find to if an array contains a specific element- array-ex- 32 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if an array contains a specific element using includes() and returns a boolean.
- Write a JavaScript function that returns the index of a given element if it exists, or -1 if it does not.
- Write a JavaScript function that iterates through an array to find and return true if a particular element is found.
- Write a JavaScript function that searches an array of objects for an object with a specific property value.
Go to:
PREV : Remove Specific Element.
NEXT : Empty Array.
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.