JavaScript: Filter false, null, 0 and blank values from an array
JavaScript Array: Exercise-39 with Solution
Filter Array Values
Write a JavaScript function to filter false, null, 0 and blank values from an array.
Test Data :
console.log(filter_array_values([58, '', 'abcd', true, null, false, 0]));
[58, "abcd", true]
Visual Presentation:
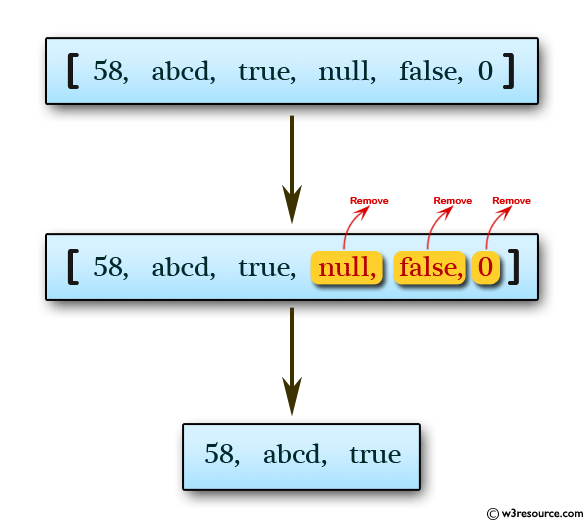
Sample Solution:
JavaScript Code:
// Function to filter array values based on eligibility criteria
function filter_array_values(arr) {
// Use the filter function with the custom eligibility check provided by 'isEligible'
arr = arr.filter(isEligible);
// Return the filtered array
return arr;
}
// Function to check the eligibility of a value
function isEligible(value) {
// Check if the value is not equal to false, null, 0, or an empty string
if (value !== false || value !== null || value !== 0 || value !== "") {
// If eligible, return the value
return value;
}
}
// Output the result of filtering the array with the custom eligibility check
console.log(filter_array_values([58, '', 'abcd', true, null, false, 0]));
Output:
[58,"abcd",true]
Flowchart:
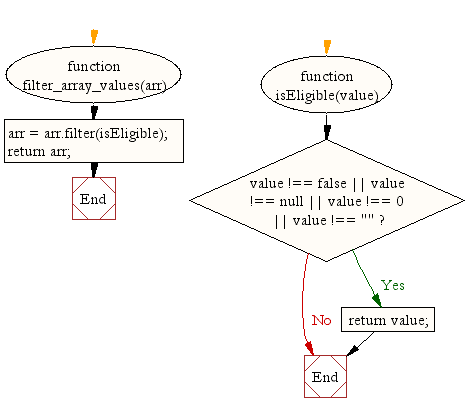
ES6 Version:
// Function to filter array values based on eligibility criteria
const filter_array_values = (arr) => {
// Use the filter function with the custom eligibility check provided by 'isEligible'
arr = arr.filter(isEligible);
// Return the filtered array
return arr;
};
// Function to check the eligibility of a value
const isEligible = (value) => {
// Check if the value is not equal to false, null, 0, or an empty string
if (value !== false || value !== null || value !== 0 || value !== "") {
// If eligible, return the value
return value;
}
};
// Output the result of filtering the array with the custom eligibility check
console.log(filter_array_values([58, '', 'abcd', true, null, false, 0]));
Live Demo:
See the Pen JavaScript - Filter false, null, 0 and blank values from an array-array-ex- 39 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that filters false, null, 0, "", undefined, and NaN values from an array using filter().
- Write a JavaScript function that returns a new array containing only truthy values from the original array.
- Write a JavaScript function that manually iterates over an array to remove any falsy values.
- Write a JavaScript function that validates the array input and ensures the result contains only truthy elements.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to move an array element from one position to another.
Next: Write a JavaScript function to generate an array of specified length, filled with integer numbers, increase by one from starting position.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.