JavaScript: Divide an integer by another integer as long as the result is an integer and return the result
JavaScript Basic: Exercise-106 with Solution
Divide Integers Until Result is Integer
Write a JavaScript program to divide an integer by another integer as long as the result is an integer and return the result.
Sample Solution:
JavaScript Code:
// Function to divide a number by a digit
function divide_digit(num, d) {
// Check if divisor is 1, return the number itself
if (d == 1)
return num;
else {
// Loop to divide the number by the divisor until not divisible
while (num % d === 0) {
num /= d; // Divide the number by the divisor
}
return num; // Return the final divided number
}
}
// Example usage of the function with different numbers and divisors
console.log(divide_digit(-12, 2)); // Output: -3
console.log(divide_digit(13, 2)); // Output: 13
console.log(divide_digit(13, 1)); // Output: 13
Output:
-3 13 13
Live Demo:
See the Pen javascript-basic-exercise-106 by w3resource (@w3resource) on CodePen.
Flowchart:
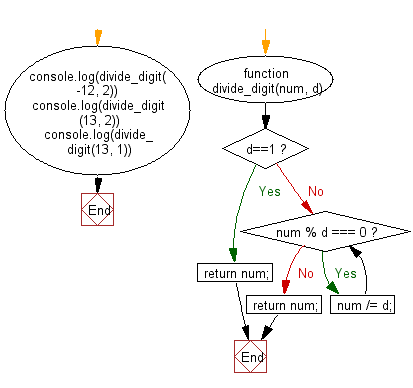
ES6 Version:
// Function to divide a number by a divisor until it's not divisible anymore
const divide_digit = (num, d) => {
if (d === 1) // If the divisor is 1, return the number as it is
return num;
else {
while (num % d === 0) { // Loop while the number is divisible by the divisor
num /= d; // Divide the number by the divisor
}
return num; // Return the resulting number after division
}
};
// Example usage of the function
console.log(divide_digit(-12, 2)); // Output: -3 (divided -12 by 2 until it's not divisible)
console.log(divide_digit(13, 2)); // Output: 13 (13 is not divisible by 2)
console.log(divide_digit(13, 1)); // Output: 13 (divisor is 1, returns the number as it is)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that continuously divides one integer by another as long as the quotient remains an integer.
- Write a JavaScript function that checks divisibility at each step and stops when further division would result in a non-integer value.
- Write a JavaScript program that performs repeated integer division and returns the final result, ensuring the quotient remains whole at every step.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the number of times to replace a given number with the sum of its digits until it convert to a single digit number.
Next: JavaScript program to find the number of sorted pairs formed by its elements of an given array of integers such that one element in the pair is divisible by the other one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.