JavaScript: Check whether a point lies strictly inside a given circle
JavaScript Basic: Exercise-120 with Solution
Check if Point is Inside Circle
Write a JavaScript program to check if a point lies strictly inside the circle.
Input:
Center of the circle (x, y)
Radius of circle: r
Point inside a circle (a, b)
Sample Solution:
JavaScript Code:
// Function to check if a point (a, b) is inside a circle with center (x, y) and radius r
function check_a_point(a, b, x, y, r) {
// Calculate the squared distance between the center of the circle (x, y) and the point (a, b)
var dist_points = (a - x) * (a - x) + (b - y) * (b - y);
// Square the radius for comparison
r *= r;
// Check if the squared distance between points is less than the squared radius
if (dist_points < r) {
return true; // Point is inside the circle
}
return false; // Point is outside the circle or on the circle
}
// Test cases
console.log(check_a_point(0, 0, 2, 4, 6)); // Output: true (Point (0,0) is inside the circle with center (2,4) and radius 6)
console.log(check_a_point(0, 0, 6, 8, 6)); // Output: false (Point (0,0) is outside the circle with center (6,8) and radius 6)
Output:
true false
Live Demo:
See the Pen javascript-basic-exercise-120 by w3resource (@w3resource) on CodePen.
Flowchart:
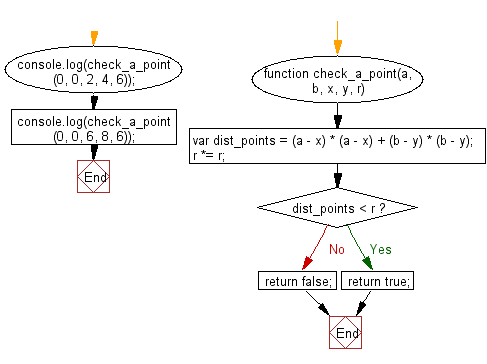
ES6 Version:
// Function to check if a point (a, b) is inside a circle with center (x, y) and radius r
const check_a_point = (a, b, x, y, r) => {
// Calculate the squared distance between the center of the circle (x, y) and the point (a, b)
const dist_points = (a - x) ** 2 + (b - y) ** 2;
// Square the radius for comparison
r **= 2;
// Check if the squared distance between points is less than the squared radius
if (dist_points < r) {
return true; // Point is inside the circle
}
return false; // Point is outside the circle or on the circle
}
// Test cases
console.log(check_a_point(0, 0, 2, 4, 6)); // Output: true (Point (0,0) is inside the circle with center (2,4) and radius 6)
console.log(check_a_point(0, 0, 6, 8, 6)); // Output: false (Point (0,0) is outside the circle with center (6,8) and radius 6)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that determines if a given point lies strictly inside a circle using the distance formula.
- Write a JavaScript function that calculates the distance between a point and a circle’s center and checks it against the radius.
- Write a JavaScript program that validates input coordinates and returns true if the point is inside the circle, false otherwise.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a given integer has an increasing digits sequence.
Next: JavaScript program to check whether a given matrix is lower triangular or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.