JavaScript: Create an array of prefix sums of the given array
JavaScript Basic: Exercise-131 with Solution
Create Prefix Sum Array
Write a JavaScript program to create an array of prefix sums of the given array.
In computer science, the prefix sum, cumulative sum, inclusive scan, or simply scan of a sequence of numbers x0, x1, x2, ... is a second sequence of numbers y0, y1, y2, ..., the sums of prefixes of the input sequence:
y0 = x0
y1 = x0 + x1
y2 = x0 + x1+ x2
...
Sample Solution:
JavaScript Code:
// Function to compute prefix sums of an array
function prefix_sums(arr) {
var new_arr = []; // Initialize an empty array to store prefix sums
// Loop through the input array
for (var i = 0; i < arr.length; i++) {
new_arr[i] = 0; // Initialize each element in the new array to 0
// Compute the prefix sum up to index 'i' in the input array
for (var j = 0; j < i + 1; j++) {
new_arr[i] += arr[j]; // Sum the elements from index 0 to 'i'
}
}
return new_arr; // Return the array containing prefix sums
}
// Test cases
console.log(prefix_sums([1, 2, 3, 4, 5])); // Output: [1, 3, 6, 10, 15]
console.log(prefix_sums([1, 2, -3, 4, 5])); // Output: [1, 3, 0, 4, 9]
Output:
[1,3,6,10,15] [1,3,0,4,9]
Live Demo:
See the Pen javascript-basic-exercise-131 by w3resource (@w3resource) on CodePen.
Flowchart:
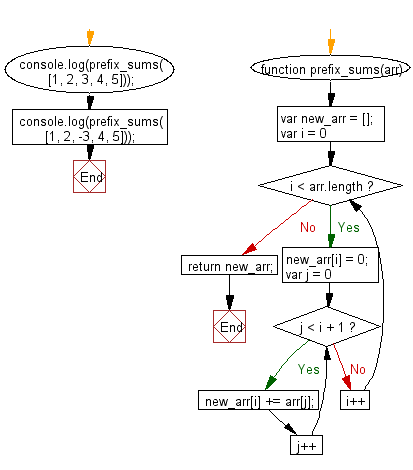
ES6 Version:
// Function to compute prefix sums of an array
const prefix_sums = (arr) => {
const new_arr = []; // Initialize an empty array to store prefix sums
// Loop through the input array
for (let i = 0; i < arr.length; i++) {
new_arr[i] = 0; // Initialize each element in the new array to 0
// Compute the prefix sum up to index 'i' in the input array
for (let j = 0; j < i + 1; j++) {
new_arr[i] += arr[j]; // Sum the elements from index 0 to 'i'
}
}
return new_arr; // Return the array containing prefix sums
}
// Test cases
console.log(prefix_sums([1, 2, 3, 4, 5])); // Output: [1, 3, 6, 10, 15]
console.log(prefix_sums([1, 2, -3, 4, 5])); // Output: [1, 3, 0, 4, 9]
For more Practice: Solve these Related Problems:
- Write a JavaScript program that generates a prefix sum array from a given array of integers using iterative addition.
- Write a JavaScript function that transforms an input array into an array of cumulative sums, handling empty arrays appropriately.
- Write a JavaScript program that uses the reduce() method to build a prefix sum array and outputs intermediate cumulative totals.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find the smallest prime number strictly greater than a given number.
Next: JavaScript program to find all distinct prime factors of a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.