JavaScript: Test whether a given integer is greater than 15 return the given number, otherwise return 15
JavaScript Basic: Exercise-137 with Solution
Return Number if >15, Else 15
Write a JavaScript program to test whether a given integer is greater than 15 and return the given number, otherwise return 15.
Visual Presentation:
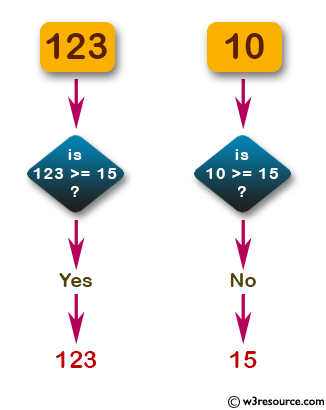
Sample Solution:
JavaScript Code:
/**
* Function to increment 'num' until it reaches or exceeds 15
* @param {number} num - The input number
* @returns {number} - The updated number (num >= 15)
*/
function test_fifteen(num) {
while (num < 15) { // Loop continues until 'num' reaches or exceeds 15
num++; // Increment 'num' by 1
}
return num; // Return the updated 'num'
}
console.log(test_fifteen("123")); // Output: 123 (input not converted to a number)
console.log(test_fifteen("10")); // Output: 15 (input incremented until 15)
console.log(test_fifteen("5")); // Output: 15 (input incremented until 15)
Output:
123 15 15
Live Demo:
See the Pen javascript-basic-exercise-137 by w3resource (@w3resource) on CodePen.
Flowchart:
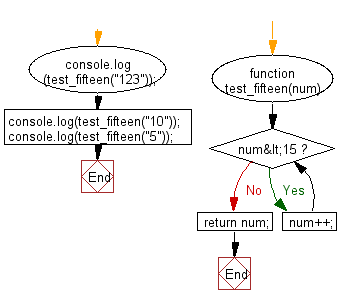
ES6 Version:
/**
* Function to increment 'num' until it reaches or exceeds 15
* @param {number} num - The input number
* @returns {number} - The updated number (num >= 15)
*/
const test_fifteen = (num) => {
while (num < 15) { // Loop continues until 'num' reaches or exceeds 15
num++; // Increment 'num' by 1
}
return num; // Return the updated 'num'
}
console.log(test_fifteen("123")); // Output: 123 (input not converted to a number)
console.log(test_fifteen("10")); // Output: 15 (input incremented until 15)
console.log(test_fifteen("5")); // Output: 15 (input incremented until 15)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if an input integer is greater than 15 and returns the number if true, otherwise returns 15.
- Write a JavaScript function that compares an integer to 15 and conditionally returns either the input number or the constant 15.
- Write a JavaScript program that validates a number and returns it if it exceeds 15, defaulting to 15 otherwise.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to replace the first digit in a string (should contains at least digit) with $ character.
Next: JavaScript program to reverse the bits of a given 16 bits unsigned short integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.