JavaScript: Change the capitalization of all letters in a given string
JavaScript Basic: Exercise-149 with Solution
Change Capitalization of All Letters
Write a JavaScript program to change the capitalization of all letters in a given string.
Visual Presentation:
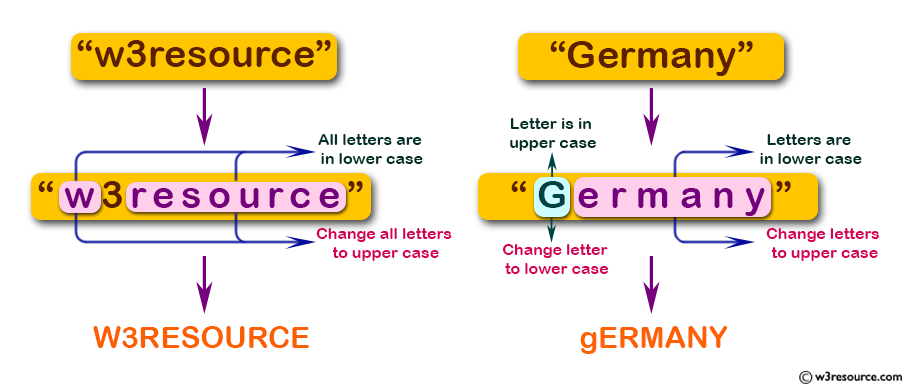
Sample Solution:
JavaScript Code:
/**
* Function to change the case of characters in a string
* @param {string} txt - The input string
* @returns {string} - The string with changed case characters
*/
function change_case(txt) {
var str1 = "";
// Loop through each character in the input string
for (var i = 0; i < txt.length; i++) {
// Check if the character is uppercase, change to lowercase; otherwise, change to uppercase
if (/[A-Z]/.test(txt[i])) str1 += txt[i].toLowerCase();
else str1 += txt[i].toUpperCase();
}
return str1; // Return the modified string
}
// Test cases
console.log(change_case("w3resource")); // Output: W3RESOURCE
console.log(change_case("Germany")); // Output: gERMANY
Output:
W3RESOURCE gERMANY
Live Demo:
See the Pen javascript-basic-exercise-149 by w3resource (@w3resource) on CodePen.
Flowchart:
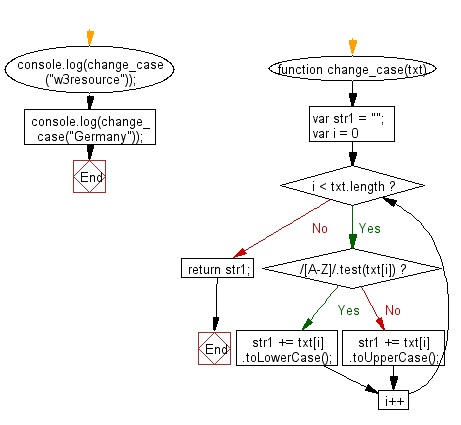
ES6 Version:
/**
* Function to change the case of characters in a string
* @param {string} txt - The input string
* @returns {string} - The string with changed case characters
*/
const change_case = (txt) => {
let str1 = "";
// Loop through each character in the input string
for (let i = 0; i < txt.length; i++) {
// Check if the character is uppercase, change to lowercase; otherwise, change to uppercase
if (/[A-Z]/.test(txt[i])) str1 += txt[i].toLowerCase();
else str1 += txt[i].toUpperCase();
}
return str1; // Return the modified string
}
// Test cases
console.log(change_case("w3resource")); // Output: W3RESOURCE
console.log(change_case("Germany")); // Output: gERMANY
For more Practice: Solve these Related Problems:
- Write a JavaScript program that toggles the case of each letter in a string, converting uppercase letters to lowercase and vice versa.
- Write a JavaScript function that iterates over a string and changes the capitalization of every character, preserving non-letter characters.
- Write a JavaScript program that transforms a string by inverting the case of each letter using character code manipulation.
Go to:
PREV : Swap Halves of Even-Length Array.
NEXT : Swap Adjacent Pairs in Even-Length Integer.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.