JavaScript: Check whether two given integer values are in the range 50..99
JavaScript Basic: Exercise-28 with Solution
Check if Two Integers are in Range 50–99
Write a JavaScript program to check whether two given integer values are in the range 50..99 (inclusive). Return true if either of them falls within the range.
This JavaScript program checks if either of two given integers falls within the range of 50 to 99 (inclusive). It uses conditional statements to evaluate whether each integer is within the specified range and returns true if at least one of them meets the condition.
Visual Presentation:
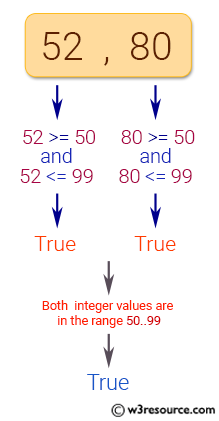
Sample Solution:
JavaScript Code:
// Define a function named check_numbers that takes two parameters x and y
function check_numbers(x, y) {
// Check if x or y is in the range between 50 and 99 (inclusive)
if ((x >= 50 && x <= 99) || (y >= 50 && y <= 99)) {
// If true, return true
return true;
} else {
// If not in the specified range, return false
return false;
}
}
// Log the result of calling the check_numbers function with the arguments 12 and 101 to the console
console.log(check_numbers(12, 101));
// Log the result of calling the check_numbers function with the arguments 52 and 80 to the console
console.log(check_numbers(52, 80));
// Log the result of calling the check_numbers function with the arguments 15 and 99 to the console
console.log(check_numbers(15, 99));
Output:
false true true
Live Demo:
See the Pen JavaScript: check if two given integer values are in a range-basic- ex-28 by w3resource (@w3resource) on CodePen.
Flowchart:
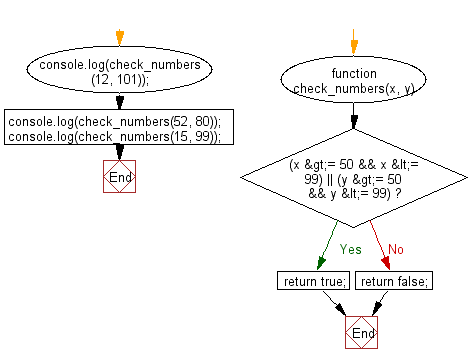
ES6 Version:
// Define a function named check_numbers using arrow function syntax
const check_numbers = (x, y) => {
// Check if x or y is in the range between 50 and 99 (inclusive)
return (x >= 50 && x <= 99) || (y >= 50 && y <= 99);
};
// Log the result of calling the check_numbers function with the arguments 12 and 101 to the console
console.log(check_numbers(12, 101));
// Log the result of calling the check_numbers function with the arguments 52 and 80 to the console
console.log(check_numbers(52, 80));
// Log the result of calling the check_numbers function with the arguments 15 and 99 to the console
console.log(check_numbers(15, 99));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if both given integers fall within the range 50 to 99 (inclusive).
- Write a JavaScript program that verifies if at least one of two provided numbers is within the range 50..99.
- Write a JavaScript program that accepts two integers and returns a message indicating which one, if any, is within the 50 to 99 range.
Go to:
PREV : Check if String Starts with 'Java'.
NEXT : Check if Three Integers are in Range 50–99.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.