JavaScript: Check the total marks of a student in various examinations
JavaScript Basic: Exercise-38 with Solution
Evaluate Grades Based on Total Marks and Final Exam
Write a JavaScript program to evaluate a student's total marks across various examinations and determine their grade. The grading criteria are as follows:
- If the total marks fall within the range of 89 to 100 (inclusive), the student receives an A+ grade.
- If the examination is labeled as "Final-exam," the student will receive an A+ grade only if their total marks are 90 or greater. Assume that final examination means we pass 'true' as second parameter otherwise blank.
- If the student achieves an A+ grade, the program should return 'true'; otherwise, it should return 'false'.
Sample Solution:
JavaScript Code:
// Define a function named exam_status with parameters totmarks and is_exam
function exam_status(totmarks, is_exam) {
// Check if is_exam is truthy (evaluates to true)
if (is_exam) {
// Return true if totmarks is greater than or equal to 90
return totmarks >= 90;
}
// Return true if totmarks is between 89 and 100 (inclusive)
return totmarks >= 89 && totmarks <= 100;
}
// Log the result of calling exam_status with arguments "78" and " " to the console
console.log(exam_status("78", " "));
// Log the result of calling exam_status with arguments "89" and "true " to the console
console.log(exam_status("89", "true "));
// Log the result of calling exam_status with arguments "99" and "true " to the console
console.log(exam_status("99", "true "));
Output:
false false true
Live Demo:
See the Pen JavaScript: total marks of a student - basic-ex-38 by w3resource (@w3resource) on CodePen.
Flowchart:
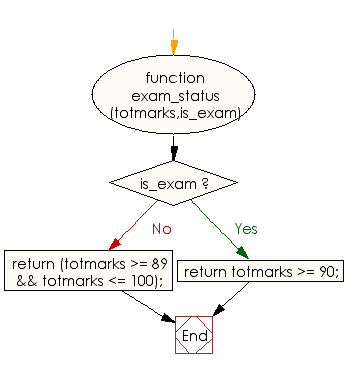
ES6 Version:
// Define a function named exam_status using arrow function syntax with parameters totmarks and is_exam
const exam_status = (totmarks, is_exam) => {
// Check if is_exam is truthy (equivalent to true)
if (is_exam) {
// Return true if totmarks is greater than or equal to 90
return totmarks >= 90;
}
// Return true if totmarks is greater than or equal to 89 and less than or equal to 100
return totmarks >= 89 && totmarks <= 100;
};
// Log the result of calling exam_status with the arguments "78" and " " to the console
console.log(exam_status("78", " "));
// Log the result of calling exam_status with the arguments "89" and "true " to the console
console.log(exam_status("89", "true "));
// Log the result of calling exam_status with the arguments "99" and "true " to the console
console.log(exam_status("99", "true "));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that calculates a student's grade based on weighted marks and returns true if the score exceeds a set threshold.
- Write a JavaScript program that evaluates total marks and a final exam score to assign an A+ grade based on custom criteria.
- Write a JavaScript program that accepts marks from multiple assessments and returns true if the final exam score meets the A+ criteria considering overall marks.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a new string with first 3 characters are in lower case from a given string.
Next: JavaScript program to compute the sum of the two given integers, If the sum is in the range 50..80 return 65 other wise return 80.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.