JavaScript: Check from two given non-negative integers that whether one of the number is multiple of 7 or 11
JavaScript Basic: Exercise-46 with Solution
Check if Only One Integer is Multiple of 7 or 11
Write a JavaScript program to check two given non-negative integers if one (not both) is a multiple of 7 or 11.
This JavaScript program examines two non-negative integers to determine if exactly one of them, but not both, is a multiple of 7 or 11. If only one of them satisfies this condition, it returns true; otherwise, it returns false.
Visual Presentation:
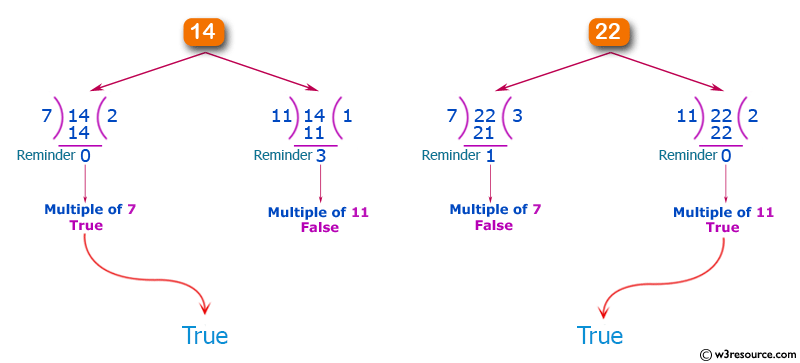
Sample Solution:
JavaScript Code:
// Define a function named valCheck with parameters a and b
function valCheck(a, b) {
// Check if the negation of the condition is true
if (!((a % 7 == 0 || a % 11 == 0) && (b % 7 == 0 || b % 11 == 0))) {
// Return true if either a is divisible by 7 or 11, or b is divisible by 7 or 11
return ((a % 7 == 0 || a % 11 == 0) || (b % 7 == 0 || b % 11 == 0));
}
else {
// If the negation of the condition is false, return false
return false;
}
}
// Log the result of calling valCheck with the arguments 14 and 21 to the console
console.log(valCheck(14, 21));
// Log the result of calling valCheck with the arguments 14 and 20 to the console
console.log(valCheck(14, 20));
// Log the result of calling valCheck with the arguments 16 and 20 to the console
console.log(valCheck(16, 20));
Output:
false true false
Live Demo:
See the Pen JavaScript: Check two given non-negative integers that whether one of the number (not both) is multiple of 7 or 11. - basic-ex-46 by w3resource (@w3resource) on CodePen.
Flowchart:
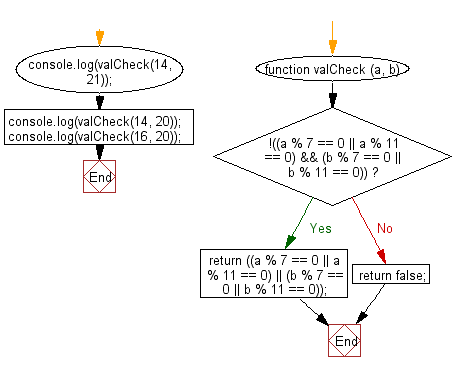
ES6 Version:
// Function to check if the numbers satisfy the given conditions
const valCheck = (a, b) => {
// Checking if neither 'a' nor 'b' satisfies the condition and returning the result
if (!((a % 7 === 0 || a % 11 === 0) && (b % 7 === 0 || b % 11 === 0))) {
return ((a % 7 === 0 || a % 11 === 0) || (b % 7 === 0 || b % 11 === 0)); // Return true if at least one number satisfies the condition
} else {
return false; // Return false if both numbers satisfy the condition
}
};
// Examples of the function usage
console.log(valCheck(14, 21)); // Output: true (Either 14 or 21 satisfy the condition)
console.log(valCheck(14, 20)); // Output: true (14 satisfies the condition)
console.log(valCheck(16, 20)); // Output: false (Neither 16 nor 20 satisfies the condition)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if exactly one out of two non-negative integers is a multiple of 7 or 11.
- Write a JavaScript program that verifies if only one of two given numbers is divisible by either 7 or 11.
- Write a JavaScript program that determines if one (but not both) of two integers is a multiple of 7 or 11 and returns a boolean.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check two given integer values and return true if one of the number is 15 or if their sum or difference is 15.
Next: JavaScript program to check if a number in the range 40..10000 presents in two number (in same range).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.