JavaScript: Convert a given number to hours and minutes
JavaScript Basic: Exercise-51 with Solution
Transform Number to Hours and Minutes
Write a JavaScript application that transforms a numerical value into hours and minutes.
This JavaScript application converts a given numerical value representing minutes into hours and minutes. It calculates the hours by dividing the total minutes by 60 and the remaining minutes using the modulus operator, then formats and displays the result.
Visual Presentation:
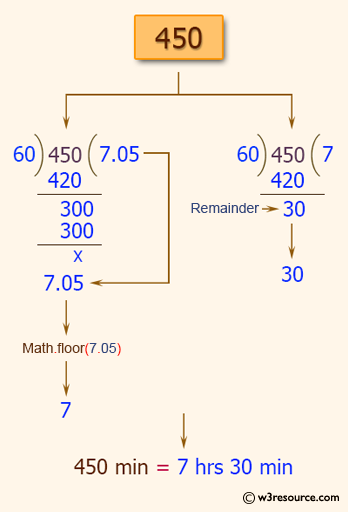
Sample Solution:
JavaScript Code:
// Define a function named time_convert with parameter num
function time_convert(num)
{
// Calculate the number of hours by dividing num by 60 and rounding down
var hours = Math.floor(num / 60);
// Calculate the remaining minutes by taking the remainder when dividing num by 60
var minutes = num % 60;
// Return the result as a string in the format "hours:minutes"
return hours + ":" + minutes;
}
// Log the result of calling time_convert with different numeric inputs to the console
console.log(time_convert(71));
console.log(time_convert(450));
console.log(time_convert(1441));
Output:
1:11 7:30 24:1
Live Demo:
See the Pen JavaScript - convert a given number to hours and minutes - basic-ex-51 by w3resource (@w3resource) on CodePen.
Flowchart:
ES6 Version:
// Define a function named time_convert with parameter num
const time_convert = (num) => {
// Calculate hours by dividing num by 60 and rounding down
const hours = Math.floor(num / 60);
// Calculate minutes by taking the remainder when num is divided by 60
const minutes = num % 60;
// Return the result as a string in the format "hours:minutes"
return `${hours}:${minutes}`;
};
// Log the result of calling time_convert with the given numbers to the console
console.log(time_convert(71));
console.log(time_convert(450));
console.log(time_convert(1441));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a total number of minutes into a string formatted as "Xh Ym", handling edge cases like negative values.
- Write a JavaScript function that transforms a given number into days, hours, and minutes when the number exceeds 1440 minutes.
- Write a JavaScript program that accepts a floating-point number representing minutes and returns hours and minutes with proper rounding and leading zeros.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to capitalize the first letter of each word of a given string.
Next: JavaScript program to convert the letters of a given string in alphabetical order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.