JavaScript: Create a new string of specified copies of a given string
JavaScript Basic: Exercise-57 with Solution
Create String of Specified Copies
Write a JavaScript program to create a new string of specified copies (positive numbers) of a given string.
This JavaScript program takes a string and a positive number as inputs, then creates a new string by repeating the given string the specified number of times. The result is a single string made up of the original string copied multiple times.
Visual Presentation:
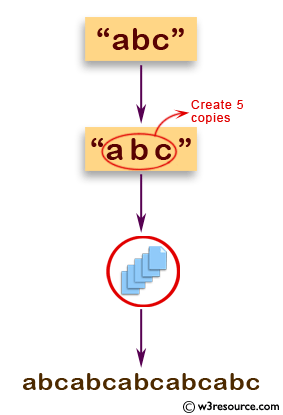
Sample Solution:
JavaScript Code:
// Define a function named string_copies with parameters str (string) and n (number)
function string_copies(str, n) {
// Check if n is less than 0
if (n < 0)
// Return false if n is negative
return false;
else
// Use the repeat method to replicate the string 'n' times
return str.repeat(n);
}
// Log the result of calling string_copies with the arguments "abc" and 5 to the console
console.log(string_copies("abc", 5));
// Log the result of calling string_copies with the arguments "abc" and 0 to the console
console.log(string_copies("abc", 0));
// Log the result of calling string_copies with the arguments "abc" and -2 to the console
console.log(string_copies("abc", -2));
Output:
abcabcabcabcabc false
Live Demo:
See the Pen JavaScript - create a new string of specified copies (positive number) of a given string. - basic-ex-57 by w3resource (@w3resource) on CodePen.
Flowchart:
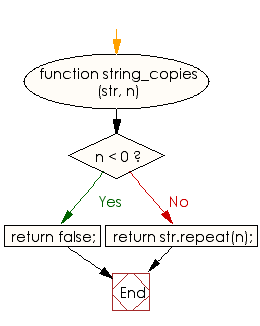
ES6 Version:
// Define a function named string_copies with parameters str and n
const string_copies = (str, n) => {
// Check if n is less than 0, and return false if true
if (n < 0) {
return false;
} else {
// Use the repeat method to repeat the string n times
return str.repeat(n);
}
};
// Call the function with sample arguments and log the results to the console
console.log(string_copies("abc", 5));
console.log(string_copies("abc", 0));
console.log(string_copies("abc", -2));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that generates a new string by repeating a given input string a specified number of times without using the built-in repeat() method.
- Write a JavaScript function that recursively constructs a string consisting of n copies of an input string.
- Write a JavaScript program that validates the number of copies before generating a repeated string, returning an error for non-positive numbers.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to divide two positive numbers and return a string with properly formatted commas.
Next: JavaScript program to create a new string of 4 copies of the last 3 characters of a given original string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.