JavaScript: Concatenate two strings and return the result
JavaScript Basic: Exercise-64 with Solution
Concatenate Strings with Matching Length
Write a JavaScript program to concatenate two strings and return the result. If the length of the strings does not match, then remove the characters from the longer string.
For example "Python" and "JS" will be "onJS".
This JavaScript program concatenates two strings and, if their lengths differ, trims the longer string to match the length of the shorter one before concatenation.
Visual Presentation:
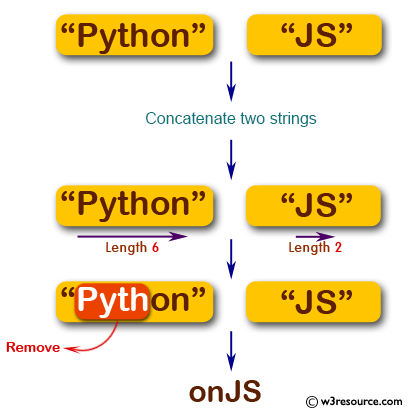
Sample Solution:
JavaScript Code:
// Define a function named str_con_cat with parameters str1 and str2
function str_con_cat(str1, str2) {
// Calculate the minimum length of str1 and str2
const m = Math.min(str1.length, str2.length);
// Use substring to concatenate the common characters from the end of both strings
return str1.substring(str1.length - m) + str2.substring(str2.length - m);
}
// Call the function with sample arguments and log the results to the console
console.log(str_con_cat("Python", "JS"));
console.log(str_con_cat("ab", "cdef"));
Output:
onJS abef
Live Demo:
See the Pen JavaScript - Concatenate two strings and return the result - basic-ex-64 by w3resource (@w3resource) on CodePen.
Flowchart:
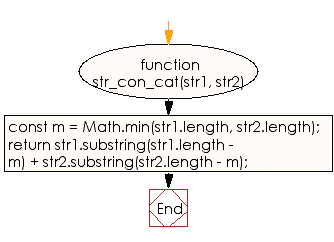
ES6 Version:
// Define a function named str_con_cat with parameters str1 and str2
const str_con_cat = (str1, str2) => {
// Use Math.min to find the minimum length between str1 and str2
const m = Math.min(str1.length, str2.length);
// Concatenate substrings using substring method and return the result
return str1.substring(str1.length - m) + str2.substring(str2.length - m);
};
// Call the function with sample arguments and log the results to the console
console.log(str_con_cat("Python", "JS"));
console.log(str_con_cat("ab", "cdef"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a string using the middle three characters of a given string of odd length.
Next: JavaScript program to test if a string end with "Script". The string length must be greater or equal to 6.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-basic-exercise-64.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics