JavaScript: Create a new array taking the first and last elements from a given array of integers and length must be greater or equal to 1
JavaScript Basic: Exercise-76 with Solution
Create Array with First/Last Elements from Array
Write a JavaScript program to create an array by taking the first and last elements from a given array of integers. The length must be larger than or equal to 1.
Visual Presentation:
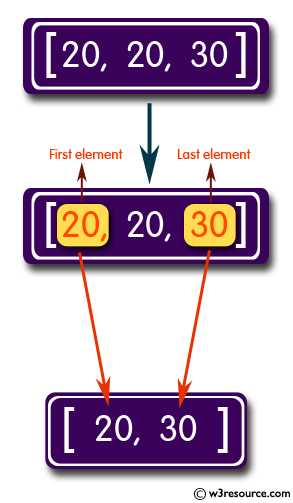
Sample Solution:
JavaScript Code:
// Function that returns an array containing the first and last elements of the input array
function started(nums) {
var array1 = []; // Create an empty array to store the first and last elements
array1.push(nums[0], nums[nums.length - 1]); // Add the first and last elements to the array
return array1; // Return the array with the first and last elements
}
// Example usage of the started function with different input arrays
console.log(started([20, 20, 30])); // Output: [20, 30]
console.log(started([5, 2, 7, 8])); // Output: [5, 8]
console.log(started([17, 12, 34, 78])); // Output: [17, 78]
Output:
[20,30] [5,8] [17,78]
Live Demo:
See the Pen JavaScript - Create a new array taking the first and last elements from a given array of integers - basic-ex-76 by w3resource (@w3resource) on CodePen.
Flowchart:
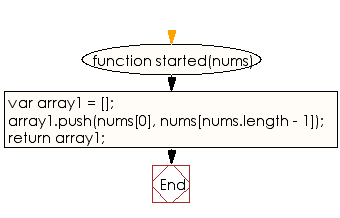
ES6 Version:
// Function that returns an array containing the first and last elements of the input array
function started(nums) {
var array1 = []; // Create an empty array to store the first and last elements
array1.push(nums[0], nums[nums.length - 1]); // Add the first and last elements to the array
return array1; // Return the array with the first and last elements
}
// Example usage of the started function with different input arrays
console.log(started([20, 20, 30])); // Output: [20, 30]
console.log(started([5, 2, 7, 8])); // Output: [5, 8]
console.log(started([17, 12, 34, 78])); // Output: [17, 78]
For more Practice: Solve these Related Problems:
- Write a JavaScript program that forms a new array by extracting the first and last elements from a given array of integers.
- Write a JavaScript function that validates an array’s length and returns an array containing only its first and last items.
- Write a JavaScript program that retrieves the boundary elements of an array and outputs them in a new array, handling arrays with one element gracefully.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to create a new array taking the middle elements of the two arrays of integer and each length 3.
Next: JavaScript program to test if an array of integers of length 2 contains 1 or a 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.