JavaScript: Check whether two given integers are similar or not
JavaScript Basic: Exercise-88 with Solution
Check Similarity of Two Integers with Divisor
Write a JavaScript program that takes two integers and a divisor. If the given divisor divides both integers and does not divide either, two specified integers are similar. Check whether two integers are similar or not.
Visual Presentation:
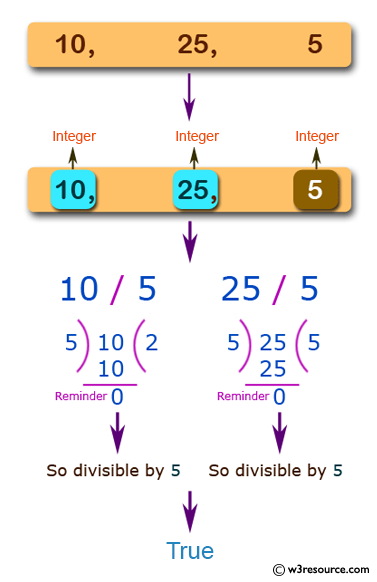
Sample Solution:
JavaScript Code:
// Function to check if two numbers are both divisible or not divisible by a given divisor
function checking_numbers(x, y, divisor) {
// Check if both x and y are divisible by the divisor or not divisible by the divisor
if (x % divisor === 0 && y % divisor === 0 || x % divisor !== 0 && y % divisor !== 0) {
return true; // Return true if the condition is satisfied
}
return false; // Return false if the condition is not satisfied
}
// Example usage
console.log(checking_numbers(10, 25, 5)); // true
console.log(checking_numbers(10, 20, 5)); // true
console.log(checking_numbers(10, 20, 4)); // false
Output:
true true false
Live Demo:
See the Pen javascript-basic-exercise-88 by w3resource (@w3resource) on CodePen.
Flowchart:
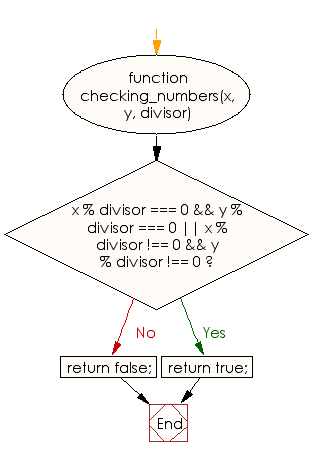
ES6 Version:
// Function to check if both numbers are divisible by the given divisor or not
const checking_numbers = (x, y, divisor) => {
// Check if both x and y are divisible by the divisor or not, or neither is divisible
if ((x % divisor === 0 && y % divisor === 0) || (x % divisor !== 0 && y % divisor !== 0)) {
return true;
}
// If not both or neither, return false
return false;
};
// Example usage
console.log(checking_numbers(10, 25, 5)); // true
console.log(checking_numbers(10, 20, 5)); // true
console.log(checking_numbers(10, 20, 4)); // false
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes two integers and a divisor, then checks if both numbers share the same divisibility status regarding that divisor.
- Write a JavaScript function that returns true if both integers are divisible by a given number or if neither is divisible, and false otherwise.
- Write a JavaScript program that accepts two numbers and a divisor, and compares their remainders to determine similarity based on divisibility.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether two arrays of integers and same length are similar or not.
Next: JavaScript program to check whether it is possible to replace $ in a given expression x $ y = z with one of the four signs +, -, * or / to obtain a correct expression.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.