JavaScript: Find the kth greatest element of a given array of integers
JavaScript Basic: Exercise-90 with Solution
Find kth Greatest Element in Array
Write a JavaScript program to find the kth greatest element in a given array of integers.
Visual Presentation:
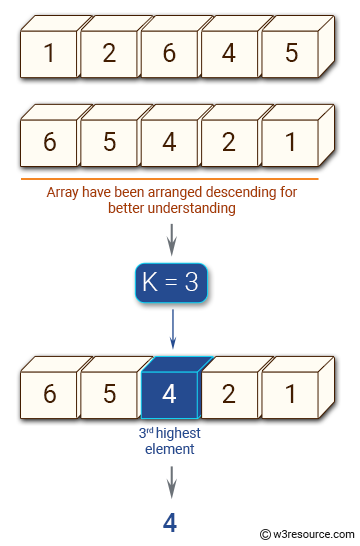
Sample Solution:
JavaScript Code:
// Function to find the Kth greatest element in an array
function Kth_greatest_in_array(arr, k) {
// Iterate for the first K elements
for (var i = 0; i < k; i++) {
var max_index = i, // Assume the current index as the maximum index
tmp = arr[i]; // Temporary variable to store the current element
// Iterate through the remaining elements to find the maximum
for (var j = i + 1; j < arr.length; j++) {
// If the current element is greater than the element at max_index, update max_index
if (arr[j] > arr[max_index]) {
max_index = j;
}
}
// Swap the current element with the maximum element found
arr[i] = arr[max_index];
arr[max_index] = tmp;
}
// Return the Kth greatest element
return arr[k - 1];
}
// Example usage
console.log(Kth_greatest_in_array([1,2,6,4,5], 3)); // 4
console.log(Kth_greatest_in_array([-10,-25,-47,-36,0], 1)); // 0
Output:
4 0
Live Demo:
See the Pen javascript-basic-exercise-90 by w3resource (@w3resource) on CodePen.
Flowchart:
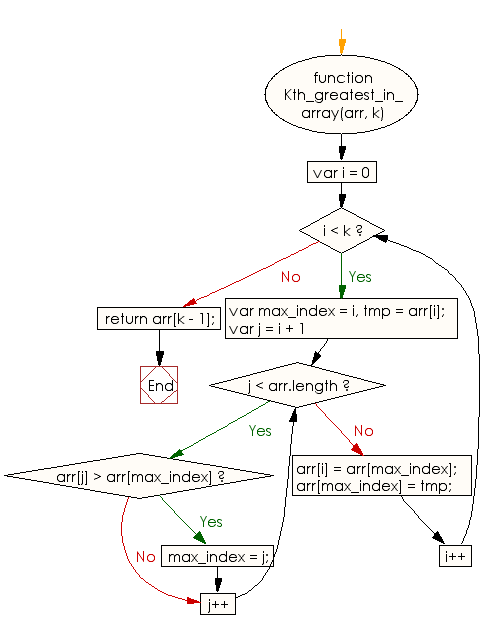
ES6 Version:
// Function to find the kth greatest element in an array
const Kth_greatest_in_array = (arr, k) => {
// Iterate up to the kth element
for (let i = 0; i < k; i++) {
// Initialize max_index and tmp for the current iteration
let max_index = i,
tmp = arr[i];
// Iterate through the remaining elements to find the maximum
for (let j = i + 1; j < arr.length; j++) {
// Update max_index if a greater element is found
if (arr[j] > arr[max_index]) {
max_index = j;
}
}
// Swap the current element with the maximum element found
arr[i] = arr[max_index];
arr[max_index] = tmp;
}
// Return the kth greatest element
return arr[k - 1];
};
// Example usage
console.log(Kth_greatest_in_array([1, 2, 6, 4, 5], 3)); // 4
console.log(Kth_greatest_in_array([-10, -25, -47, -36, 0], 1)); // 0
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the kth greatest element in an unsorted array without sorting the entire array.
- Write a JavaScript function that implements the quickselect algorithm to efficiently determine the kth largest number in an array.
- Write a JavaScript program that iterates through an array to find and return the kth greatest element using a partitioning strategy.
Go to:
PREV : Replace $ in Expression to Make True.
NEXT : Find Max Sum of k Consecutive Numbers in Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.