JavaScript: Find the sign of product of three numbers
JavaScript Conditional Statement and loops: Exercise-2 with Solution
Sign of Product of Three Numbers
Write a JavaScript conditional statement to find the sign of the product of three numbers. Display an alert box with the specified sign.
Sample numbers : 3, -7, 2
Output : The sign is -
Sample Solution-1:
JavaScript Code:
// Assigning values to variables
var x = 3;
var y = -7;
var z = 2;
// Checking different conditions based on the signs of x, y, and z
if (x > 0 && y > 0 && z > 0) {
alert("The sign is +");
} else if (x < 0 && y < 0 && z < 0) {
console.log("The sign is -");
} else if (x > 0 && y < 0 && z < 0) {
console.log("The sign is +");
} else if (x < 0 && y > 0 && z < 0) {
console.log("The sign is +");
} else {
console.log("The sign is -");
}
Output:
The sign is -
Flowchart:
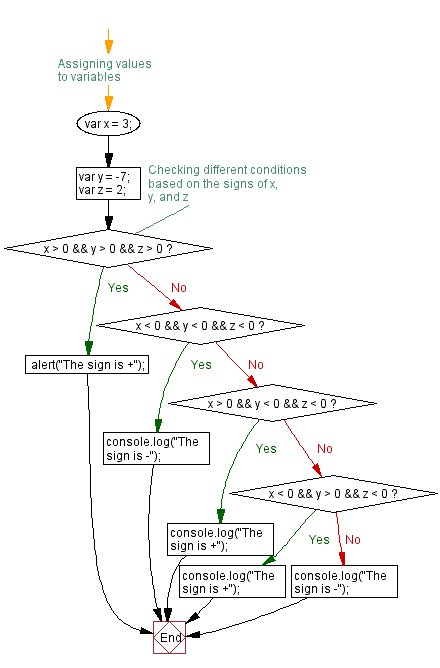
Live Demo:
See the Pen javascript-conditional-statements-and-loops-exercise-2 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Assigning values to variables
var x = 3;
var y = -7;
var z = 2;
// Function to determine the sign based on the input value
function getSign(value) {
if (value > 0) {
return "+";
} else if (value < 0) {
return "-";
} else {
return "zero";
}
}
// Checking the sign for each variable
var signX = getSign(x);
var signY = getSign(y);
var signZ = getSign(z);
// Determining the overall sign based on individual signs
var overallSign = (signX === signY && signY === signZ) ? "+" : "-";
// Displaying the result
console.log("The sign is " + overallSign);
Output:
The sign is -
Flowchart:
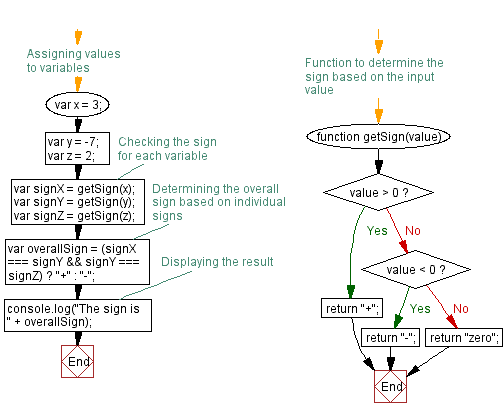
For more Practice: Solve these Related Problems:
- Write a JavaScript function that determines the sign of the product of three numbers without multiplying them directly.
- Write a JavaScript function that uses logical operators to deduce the sign of the product of three numbers, including handling zeros.
- Write a JavaScript function that calculates the sign of the product by checking the number of negative inputs without performing multiplication.
- Write a JavaScript function that determines whether the product of three numbers is positive, negative, or zero using bitwise operations.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program that accept two integers and display the larger.
Next: Write a JavaScript conditional statement to sort three numbers. Display an alert box to show the result.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.