JavaScript: Return the number of minutes in hours and minutes
JavaScript Datetime: Exercise-13 with Solution
Minutes to Hours
Write a JavaScript function that returns the number of minutes in hours and minutes.
Test Data :
console.log(timeConvert(200));
Output :
"200 minutes = 3 hour(s) and 20 minute(s)."
Sample Solution:
JavaScript Code:
// Define a JavaScript function called timeConvert with parameter n
function timeConvert(n) {
// Store the input number of minutes in a variable num
var num = n;
// Calculate the total hours by dividing the number of minutes by 60
var hours = (num / 60);
// Round down the total hours to get the number of full hours
var rhours = Math.floor(hours);
// Calculate the remaining minutes after subtracting the full hours from the total hours
var minutes = (hours - rhours) * 60;
// Round the remaining minutes to the nearest whole number
var rminutes = Math.round(minutes);
// Construct and return a string representing the conversion result
return num + " minutes = " + rhours + " hour(s) and " + rminutes + " minute(s).";
}
// Output the result of converting 200 minutes to hours and minutes
console.log(timeConvert(200));
Output:
200 minutes = 3 hour(s) and 20 minute(s).
Explanation:
In the exercise above,
- The code defines a JavaScript function named "timeConvert()" with one parameter 'n', representing the number of minutes to be converted.
- Inside the function:
- It stores the input number of minutes in a variable 'num'.
- It calculates the total hours by dividing the number of minutes by 60 and assigns it to the variable 'hours'.
- It rounds down the total hours to the nearest integer using "Math.floor()" and assigns it to the variable 'rhours'.
- It calculates the remaining minutes after subtracting the full hours from the total hours and assigns it to the variable 'minutes'.
- It rounds the remaining minutes to the nearest whole number using "Math.round()" and assigns it to the variable 'rminutes'.
- It constructs a string representing the conversion result using concatenation and returns it.
- The code then demonstrates the "timeConvert()" function by calling it with the value 200 (representing 200 minutes).
Flowchart:
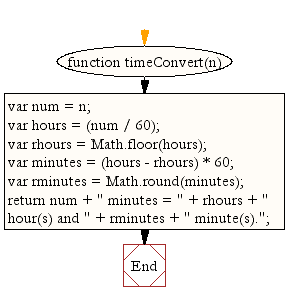
Live Demo:
See the Pen JavaScript - Return the number of minutes in hours and minutes-date-ex- 13 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a given number of minutes into hours and remaining minutes using division and modulus.
- Write a JavaScript function that returns a formatted string representing the conversion from minutes to hours and minutes.
- Write a JavaScript function that handles negative minute values by converting them appropriately.
- Write a JavaScript function that validates the input to ensure it is a positive integer before conversion.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the minimum date from an array of dates.
Next: Write a JavaScript function to get the amount of days of a year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.