JavaScript: Get ISO-8601 numeric representation of the day of the week
JavaScript Datetime: Exercise-22 with Solution
ISO Weekday Number
Write a JavaScript function to get an ISO-8601 numeric representation of the day of the week (1 (for Monday) to 7 (for Sunday).
Test Data :
dt = new Date();
console.log(ISO_numeric_date(dt));
4
dt = new Date(2015, 10, 1);
console.log(ISO_numeric_date(dt));
7
Sample Solution:
JavaScript Code:
// Define a function ISO_numeric_date that takes a Date object dt as input
function ISO_numeric_date(dt)
{
// Return the day of the week for the provided date, represented as a numeric value
// If the day is Sunday (0), return 7 instead to conform to ISO 8601 standard
return (dt.getDay() === 0 ? 7 : dt.getDay());
}
// Create a new Date object representing the current date
dt = new Date();
// Output the numeric representation of the day of the week using ISO 8601 standard for the current date
console.log(ISO_numeric_date(dt));
// Create a new Date object representing November 1, 2015
dt = new Date(2015, 10, 1);
// Output the numeric representation of the day of the week using ISO 8601 standard for November 1, 2015
console.log(ISO_numeric_date(dt));
Output:
2 7
Explanation:
In the exercise above,
- The code defines a function named "ISO_numeric_date()" that takes a Date object dt as input.
- Inside the ISO_numeric_date function:
- It checks if the day of the week for the provided date (dt.getDay()) is equal to 0, which represents Sunday.
- If the day is Sunday (0), it returns 7 instead. This adjustment is made to conform to the ISO 8601 standard, where Monday is considered the first day of the week and is represented by 1.
- After defining the function, the code creates a new Date object "dt" representing the current date using 'new Date()'. It then calls the "ISO_numeric_date()" function with this Date object and outputs the result to the console.
- Next, the code creates another new Date object "dt" representing November 1, 2015, using 'new Date(2015, 10, 1)'. It again calls the "ISO_numeric_date()" function with this Date object and outputs the result to the console.
Flowchart:
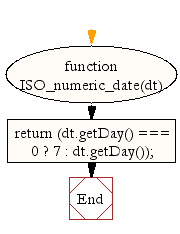
Live Demo:
See the Pen JavaScript - Get ISO-8601 numeric representation of the day of the week-date-ex-22 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the ISO-8601 numeric representation of the weekday (1 for Monday to 7 for Sunday) from a Date object.
- Write a JavaScript function that adjusts the standard getDay() value to conform to the ISO standard.
- Write a JavaScript function that converts a date string to a Date object and returns its ISO weekday number.
- Write a JavaScript function that validates the date input and returns a default value if the date is invalid.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get a full textual representation of the day of the week (Sunday through Saturday).
Next: Write a JavaScript function to get English ordinal suffix for the day of the month, 2 characters (st, nd, rd or th.).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.