JavaScript: Get a two digit representation of a year
JavaScript Datetime: Exercise-29 with Solution
Two-Digit Year
Write a JavaScript function to get a two-digit year representation.
Test Data:
dt = new Date();
console.log(sort_year(dt));
18
dt = new Date(1989, 10, 1);
console.log(sort_year(dt));
89
Sample Solution:
JavaScript Code:
// Define a JavaScript function called sort_year with parameter dt (date)
function sort_year(dt)
{
// Convert the full year value of the provided date to a string and extract the last two digits
return ('' + dt.getFullYear()).substr(2);
}
// Create a new Date object representing the current date
dt = new Date();
// Output the last two digits of the full year value for the current date
console.log(sort_year(dt));
// Create a new Date object representing November 1, 1989
dt = new Date(1989, 10, 1);
// Output the last two digits of the full year value for November 1, 1989
console.log(sort_year(dt));
Output:
18 89
Explanation:
In the exercise above,
- The code defines a JavaScript function named "sort_year()" with one parameter 'dt', representing a Date object.
- Inside the sort_year function:
- It converts the full year value of the provided Date object "dt" to a string using the "getFullYear()" method.
- It then extracts the last two digits of the string representation of the full year using the "substr()" method.
- The code then demonstrates the usage of the "sort_year()" function:
- It creates a new Date object "dt" representing the current date using 'new Date()'.
- It outputs the last two digits of the full year value for the current date by calling the "sort_year()" function with 'dt' and logging the result to the console.
- It creates another new Date object "dt" representing November 1, 1989, using 'new Date(1989, 10, 1)'.
- It outputs the last two digits of the full year value for November 1, 1989, by calling the "sort_year()" function with 'dt' and logging the result to the console.
Flowchart:
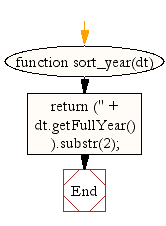
Live Demo:
See the Pen JavaScript - Get a two digit representation of a year-date-ex-29 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get a full numeric representation of a year (4 digits).
Next: Write a JavaScript function to get lowercase Ante meridiem and Post meridiem.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-date-exercise-29.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics