JavaScript: Identify a day based on a date
JavaScript Datetime: Exercise-57 with Solution
Day Name from Date String
Write a JavaScript program to get the name of a day based on a given date in string format.
Date format: mm/dd/yyyy
Test Data:
("07/11/2000") -> "Tuesday"
("11/06/2017") -> "Sunday"
("11/26/2017") -> "Not a valid Date!"
Sample Solution:
JavaScript Code:
// Define an array containing names of days of the week
const days_Name = ['Saturday', 'Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday']
// Define a function to determine the day of the week from a given date string
const Date_To_Day = (dt) => {
// Check if the input argument is a string
if (typeof dt !== 'string') {
return 'Argument should be string!'
}
// Extract the day, month, and year from the date string
let [day, month, year] = dt.split('/').map((x) => Number(x))
// Check if the date is valid
if (day < 1 || day > 31 || month > 12 || month < 1) {
return 'Not a valid Date!'
}
// Adjust month and year for calculation
if (month < 3) {
year--
month += 12
}
// Calculate the day of the week using Zeller's Congruence formula
const year_Digits = year % 100
const century = Math.floor(year / 100)
const week_Day = (day + Math.floor((month + 1) * 2.6) + year_Digits + Math.floor(year_Digits / 4) + Math.floor(century / 4) + 5 * century) % 7
// Return the name of the day corresponding to the calculated day of the week
return days_Name[week_Day]
}
// Test the function with different date strings and output the result
console.log(Date_To_Day("07/11/2000"))
console.log(Date_To_Day("11/06/2017"))
console.log(Date_To_Day("11/26/2017"))
Output:
Tuesday Sunday Not a valid Date!
Explanation:
In the exercise above,
The code defines a function "Date_To_Day()" that takes a date string in the format "MM/DD/YYYY" as input and returns the corresponding day of the week. The function calculates the day of the week based on the provided date. It first validates the input date string, extracts the day, month, and year, adjusts the month and year if necessary, and then applies the formula to determine the day of the week. Finally, it returns the name of the day (e.g., "Sunday", "Monday") based on the calculated day of the week.
Flowchart:
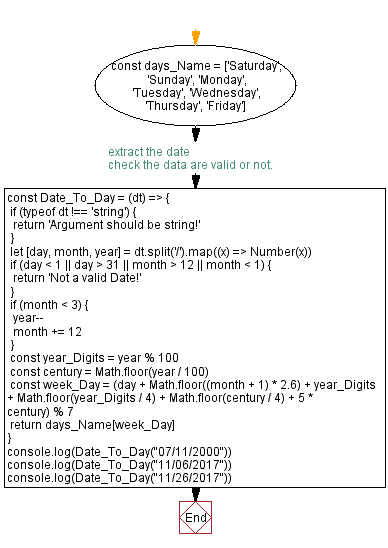
Live Demo:
See the Pen javascript-date-exercise-57 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that parses a date string and returns the full day name (e.g., "Tuesday") using a lookup array.
- Write a JavaScript function that validates a date string and returns "Not a valid Date!" if the input cannot be parsed.
- Write a JavaScript function that supports multiple date formats and returns the corresponding weekday name.
- Write a JavaScript function that converts a date string to a Date object and then outputs the day name in title case.
Improve this sample solution and post your code through Disqus.
Previous: Create and print a calendar.
Next: javascript String Exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.