JavaScript: Difference between two dates in days
JavaScript Datetime: Exercise-8 with Solution
Date Difference in Days
Write a JavaScript function to get the difference between two dates in days.
Test Data:
console.log(date_diff_indays('04/02/2014', '11/04/2014'));
console.log(date_diff_indays('12/02/2014', '11/04/2014'));
Output :
216
-28
Sample Solution:
JavaScript Code:
// Define a JavaScript function called date_diff_indays with parameters date1 and date2
var date_diff_indays = function(date1, date2) {
// Create a new Date object representing the first date
dt1 = new Date(date1);
// Create a new Date object representing the second date
dt2 = new Date(date2);
// Calculate the difference in days between the two dates
return Math.floor((Date.UTC(dt2.getFullYear(), dt2.getMonth(), dt2.getDate()) - Date.UTC(dt1.getFullYear(), dt1.getMonth(), dt1.getDate())) / (1000 * 60 * 60 * 24));
}
// Output the difference in days between '04/02/2014' and '11/04/2014'
console.log(date_diff_indays('04/02/2014', '11/04/2014'));
// Output the difference in days between '12/02/2014' and '11/04/2014'
console.log(date_diff_indays('12/02/2014', '11/04/2014'));
Output:
216 -28
Explanation:
In the exercise above,
- The code defines a JavaScript function named "date_diff_indays()" with two parameters: 'date1' and 'date2', representing two date strings.
- Inside the function:
- It creates new Date objects 'dt1' and 'dt2' by parsing the provided date strings 'date1' and 'date2', respectively.
- It calculates the difference in days between the two dates using the following steps:
- It converts both dates to UTC using Date.UTC(), which returns the number of milliseconds since January 1, 1970, 00:00:00 UTC for each date.
- It then subtracts the UTC timestamp of 'dt1' from the UTC timestamp of 'dt2' to get the difference in milliseconds between the two dates.
- Finally, it divides the difference in milliseconds by the number of milliseconds in a day (1000 milliseconds 60 seconds 60 minutes * 24 hours) to obtain the difference in days. The "Math.floor()" function is used to round down the result to the nearest integer.
- The function returns the calculated difference in days.
- The code then demonstrates the "date_diff_indays()" function by calling it with two different date pairs:
- '04/02/2014' and '11/04/2014'
- '12/02/2014' and '11/04/2014'
Flowchart:
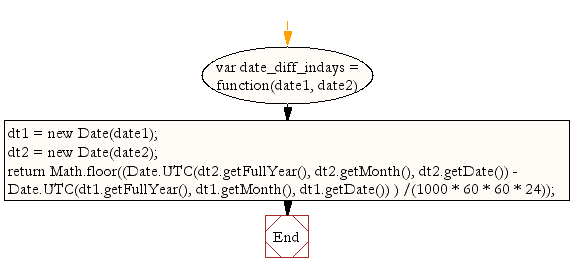
Live Demo:
See the Pen JavaScript - Difference between two dates in days-date-ex- 8 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the difference in days between two dates by subtracting their timestamps and dividing by the number of milliseconds per day.
- Write a JavaScript function that uses Math.floor to return the integer day difference between two Date objects.
- Write a JavaScript function that accepts date strings, converts them to Date objects, and returns the signed day difference.
- Write a JavaScript function that handles daylight saving time changes when computing the day difference.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to test whether a date is a weekend.
Next: Write a JavaScript function to get the last day of a month.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.