JavaScript: Set the background color of a paragraph
JavaScript DOM: Exercise-3 with Solution
Paragraph Background Color
Write a JavaScript program to set paragraph background color.
Sample Solution:
HTML Code:
<!-- Declaration of HTML document type -->
<!DOCTYPE html>
<!-- Start of HTML document -->
<html>
<!-- Start of head section -->
<head>
<!-- Declaring character encoding -->
<meta charset=utf-8 />
<!-- Setting title of the document -->
<title>Set the background color of a paragraph</title>
</head>
<!-- Start of body section -->
<body>
<!-- Button element triggering the set_background function on click -->
<input type="button" value="Click to set paragraph background color" onclick="set_background()">
<!-- First paragraph element -->
<p>w3resource JavaScript Exercises</p>
<!-- Second paragraph element -->
<p>w3resource PHP Exercises</p>
<!-- End of body section -->
</body>
<!-- End of HTML document -->
</html>
JavaScript Code:
// Function declaration for set_background
function set_background() {
// Getting reference to the body element
docBody = document.getElementsByTagName("body")[0];
// Get all the p elements that are descendants of the body
myBodyElements = docBody.getElementsByTagName("p");
// get the first p element
myp1 = myBodyElements[0];
// Setting background color for the first paragraph element
myp1.style.background = "rgb(255,0,0)";
// get the second p element
myp2 = myBodyElements[1];
// Setting background color for the second paragraph element
myp2.style.background = "rgb(255,255,0)";
}
Output:
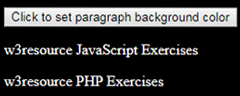
Flowchart:
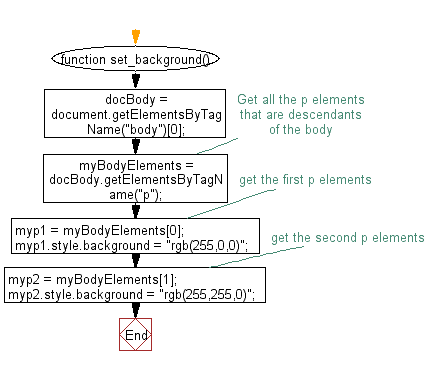
Live Demo:
See the Pen javascript-dom-exercise-3 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that cycles through an array of background colors for the paragraph each time a button is clicked.
- Write a JavaScript function that applies a random background color from a predefined palette to the paragraph on button click.
- Write a JavaScript function that changes the paragraph’s background color based on the time of day (morning, afternoon, evening).
- Write a JavaScript function that applies a CSS transition effect to smoothly change the paragraph's background color when the button is clicked.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the values of First and Last name of the following form.
Next: Write a JavaScript function to get the value of the href, hreflang, rel, target, and type attributes of the specified link.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.