JavaScript: Create a user defined table, accepting rows and columns
JavaScript DOM: Exercise-7 with Solution
Create Table Dynamically
Write a JavaScript function to create a table, accept row and column numbers, and input row-column numbers as cell content (e.g. Row-0 Column-0).
Sample HTML file:
<!DOCTYPE html>
<html>
<head>
<meta charset=utf-8 />
<title>Change the content of a cell</title>
<style type="text/css">
body {margin: 30px;}
</style>
</head><body>
<table id="myTable" border="1">
</table><form>
<input type="button" onclick="createTable()" value="Create the table">
</form></body></html>
Sample Solution:
HTML Code:
<!-- Declaration of HTML document type -->
<!DOCTYPE html>
<!-- Start of HTML document -->
<html>
<!-- Start of head section -->
<head>
<!-- Declaring character encoding -->
<meta charset=utf-8 />
<!-- Setting title of the document -->
<title>Create a table</title>
<!-- Internal CSS styling -->
<style type="text/css">
/* Styling body to have margin of 30px */
body {margin: 30px;}
</style>
<!-- End of head section -->
</head>
<!-- Start of body section -->
<body>
<!-- Table element with id 'myTable' and border attribute -->
<table id="myTable" border="1">
<!-- End of table element, currently empty -->
</table>
<!-- Form element containing a button triggering the createTable function on click -->
<form>
<input type="button" onclick="createTable()" value="Create the table">
</form>
<!-- End of body section -->
</body>
<!-- End of HTML document -->
</html>
JavaScript Code:
// Function declaration for createTable
function createTable()
{
// Prompting the user to input the number of rows
rn = window.prompt("Input number of rows", 1);
// Prompting the user to input the number of columns
cn = window.prompt("Input number of columns",1);
// Looping through rows
for(var r=0;r<parseInt(rn,10);r++)
{
// Inserting a new row at index r in the table
var x=document.getElementById('myTable').insertRow(r);
// Looping through columns
for(var c=0;c<parseInt(cn,10);c++)
{
// Inserting a new cell at index c in the current row
var y= x.insertCell(c);
// Setting the inner HTML content of the cell
y.innerHTML="Row-"+r+" Column-"+c;
}
}
}
Output:
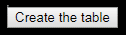
Flowchart:
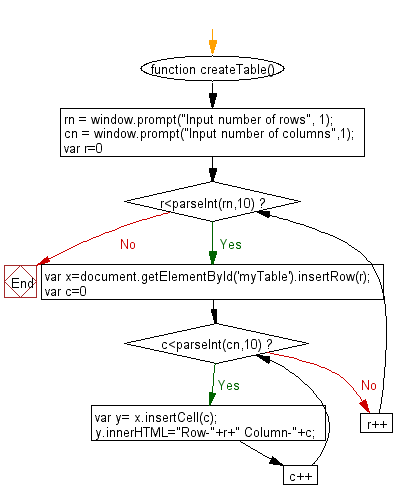
Live Demo:
See the Pen javascript-dom-exercise-7 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that creates a table with a user-specified number of rows and columns, filling each cell with its coordinates (e.g., "Row-0 Column-0").
- Write a JavaScript function that generates a table where each cell displays a random number, and appends it to the document body.
- Write a JavaScript function that builds a table dynamically and applies inline styles to each cell for visual formatting.
- Write a JavaScript function that creates a table from a two-dimensional array and renders it on the webpage.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that accept row, column, (to identify a particular cell) and a string to update the content of that cell.
Next: Write a JavaScript program to remove items from a dropdown list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.