JavaScript: Draw a circle
JavaScript Drawing: Exercise-2 with Solution
Draw Circle
Write a JavaScript program to draw a circle.
Sample output:
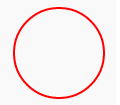
Sample Solution:
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset=utf-8 />
<title>Draw a circle</title>
</head>
<body onload="draw();">
<canvas id="circle" width="150" height="150"></canvas>
</body>
</html>
JavaScript Code:
function draw()
{
var canvas = document.getElementById('circle');
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
var X = canvas.width / 2;
var Y = canvas.height / 2;
var R = 45;
ctx.beginPath();
ctx.arc(X, Y, R, 0, 2 * Math.PI, false);
ctx.lineWidth = 3;
ctx.strokeStyle = '#FF0000';
ctx.stroke();
}
}
Live Demo:
See the Pen javascript-drawing-exercise-2 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program to draw a circle with a radial gradient that transitions from a bright center to a dark edge.
- Write a JavaScript program to draw a circle with only an outline (no fill) and a thick stroke.
- Write a JavaScript program to draw a circle with a drop shadow effect to give it a 3D appearance.
- Write a JavaScript program to draw an array of circles arranged in a grid pattern with varying colors.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to draw the following rectangular shape.
Next: Write a JavaScript program to draw two intersecting rectangles, one of which has alpha transparency.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.