JavaScript: Find the first not repeated character
JavaScript Function: Exercise-23 with Solution
Find First Non-Repeated Character
Write a JavaScript function to find the first not repeated character.
Sample arguments : 'abacddbec'
Expected output : 'e'
Visual Presentation:
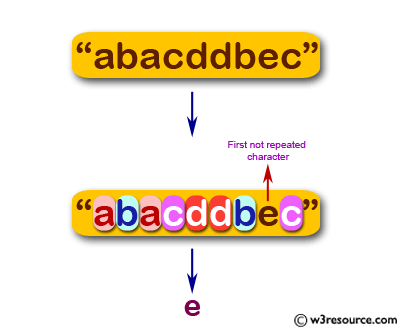
Sample Solution-1:
JavaScript Code:
// Define a function named find_FirstNotRepeatedChar that finds the first non-repeated character in a given string
function find_FirstNotRepeatedChar(str) {
// Convert the input string into an array of characters
var arra1 = str.split('');
// Initialize variables result and ctr for storing the result and counting character occurrences
var result = '';
var ctr = 0;
// Iterate through each character in the array
for (var x = 0; x < arra1.length; x++) {
// Reset the counter (ctr) for each character
ctr = 0;
// Iterate through the array again to count occurrences of the current character
for (var y = 0; y < arra1.length; y++) {
// Check if the current character is equal to the character at position y
if (arra1[x] === arra1[y]) {
// If true, increment the counter (ctr)
ctr += 1;
}
}
// Check if the counter (ctr) is less than 2 (character occurs only once)
if (ctr < 2) {
// If true, assign the current character to the result and break out of the loop
result = arra1[x];
break;
}
}
// Return the first non-repeated character found
return result;
}
// Log the result of calling find_FirstNotRepeatedChar with the input string 'abacddbec' to the console
console.log(find_FirstNotRepeatedChar('abacddbec'));
Output:
e
Flowchart:
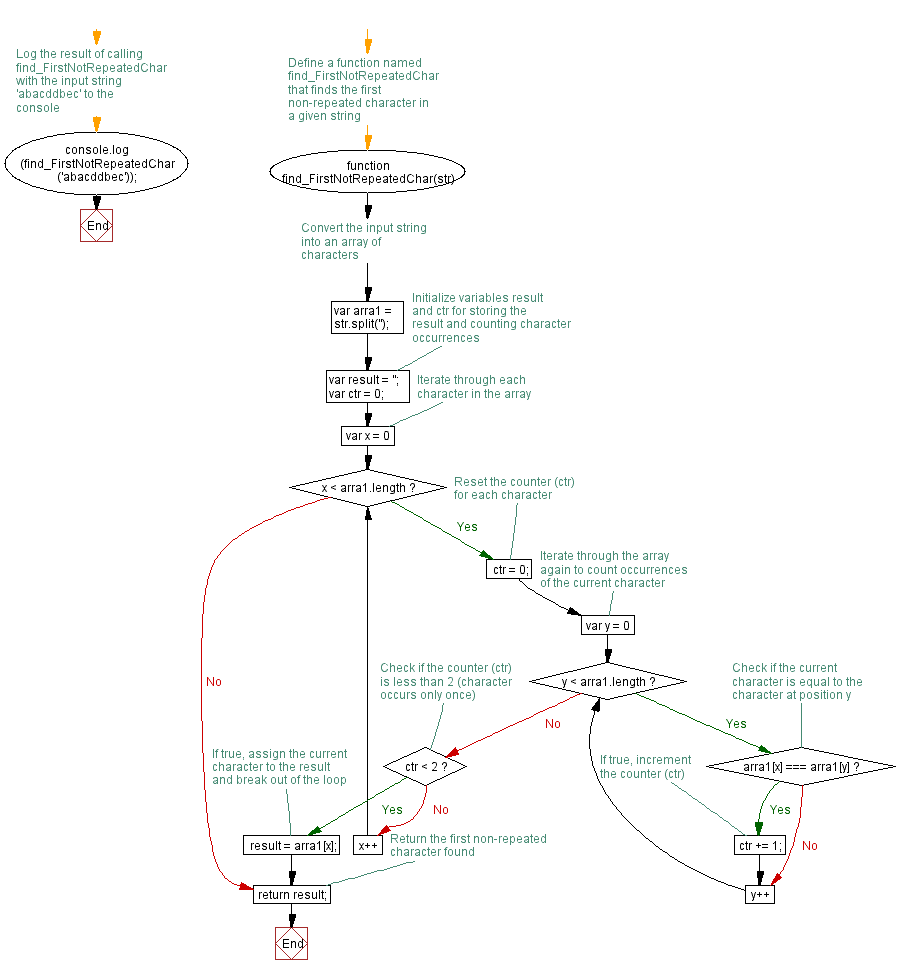
Live Demo:
See the Pen JavaScript - Find the first not repeated character-function-ex- 23 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Function to find the first non-repeated character in a string
function findFirstNotRepeatedChar(str) {
// Create a hash map to store character occurrences
var charMap = {};
// Loop through each character in the string
for (var i = 0; i < str.length; i++) {
// Use the character as a key in the hash map
var char = str[i];
// Increment the occurrence count for the current character
charMap[char] = (charMap[char] || 0) + 1;
}
// Loop through each character in the string again
for (var i = 0; i < str.length; i++) {
// If the occurrence count for the current character is 1, it is the first non-repeated character
if (charMap[str[i]] === 1) {
return str[i];
}
}
// If no non-repeated character is found, return null or a specified value
return null; // You can modify this line if you want to return a different value
}
// Example usage:
var result = findFirstNotRepeatedChar('abacddbec');
console.log(result); // Output: 'e'
Output:
e
Flowchart:
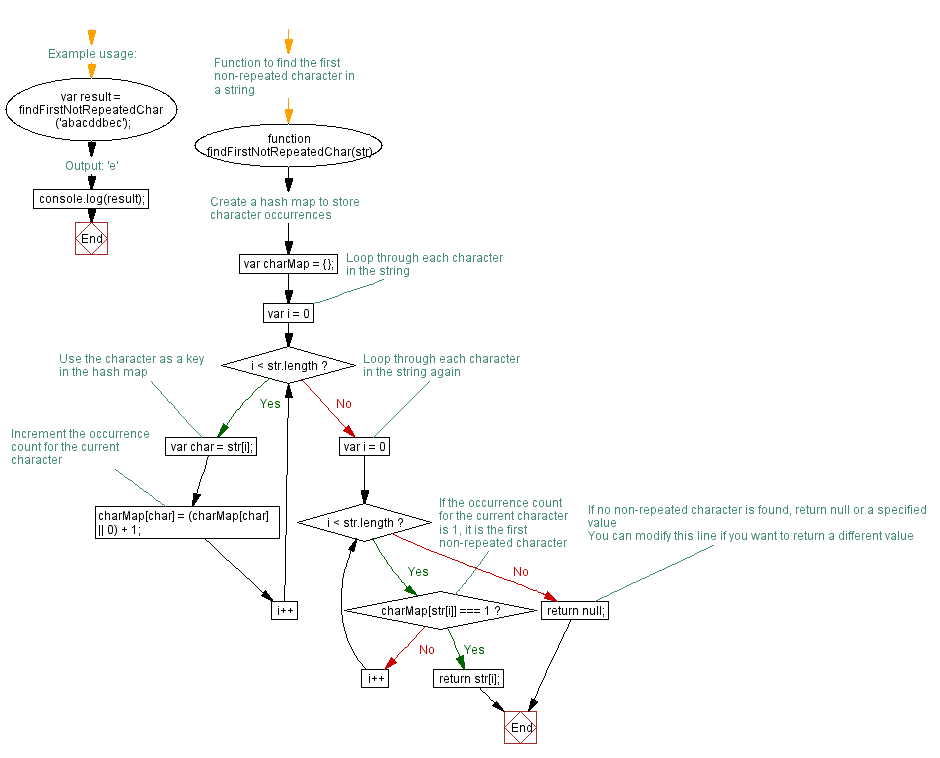
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that accepts two arguments, a string and a letter and the function will count the number of occurrences of the specified letter within the string.
Next: Write a JavaScript function to apply Bubble Sort algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-function-exercise-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics