JavaScript: The longest palindrome in a specified string
JavaScript Function: Exercise-27 with Solution
Longest Palindromic Substring
Write a JavaScript function that returns the longest palindrome in a given string.
Note: According to Wikipedia "In computer science, the longest palindromic substring or longest symmetric factor problem is
the problem of finding a maximum-length contiguous substring of a given string that is also a palindrome.
For example, the longest palindromic substring of "bananas" is "anana".
The longest palindromic substring is not guaranteed to be unique; for example,
in the string "abracadabra", there is no palindromic substring with length greater than three,
but there are two palindromic substrings with length three, namely, "aca" and "ada".
In some applications it may be necessary to return all maximal
palindromic substrings (that is, all substrings that are themselves palindromes and cannot
be extended to larger palindromic substrings) rather than returning only one substring or
returning the maximum length of a palindromic substring.
Visual Presentation:
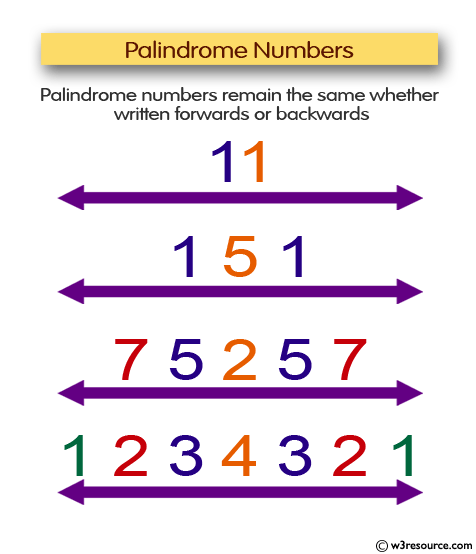
Sample Solution-1:
JavaScript Code:
// Define a function named is_Palindrome that checks if a given string is a palindrome
function is_Palindrome(str1) {
// Reverse the string and compare it with the original string
var rev = str1.split("").reverse().join("");
return str1 == rev;
}
// Define a function named longest_palindrome that finds the longest palindrome in a given string
function longest_palindrome(str1) {
// Initialize variables for maximum length (max_length) and corresponding palindrome (maxp)
var max_length = 0,
maxp = '';
// Iterate through each character in the input string
for (var i = 0; i < str1.length; i++) {
// Create a substring starting from the current character to the end of the string
var subs = str1.substr(i, str1.length);
// Iterate through each possible substring from the end of the current substring
for (var j = subs.length; j >= 0; j--) {
// Create a sub-substring from the beginning of the current substring to the current position
var sub_subs_str = subs.substr(0, j);
// Continue to the next iteration if the sub-substring has length 0 or 1
if (sub_subs_str.length <= 1)
continue;
// Check if the sub-substring is a palindrome using the is_Palindrome function
if (is_Palindrome(sub_subs_str)) {
// If true, check if it has a longer length than the current maximum length
if (sub_subs_str.length > max_length) {
// If true, update the maximum length and corresponding palindrome
max_length = sub_subs_str.length;
maxp = sub_subs_str;
}
}
}
}
// Return the longest palindrome found
return maxp;
}
// Log the result of calling longest_palindrome with the input "abracadabra" to the console
console.log(longest_palindrome("abracadabra"));
// Log the result of calling longest_palindrome with a longer input to the console
console.log(longest_palindrome("HYTBCABADEFGHABCDEDCBAGHTFYW12345678987654321ZWETYGDE"));
Output:
aca 12345678987654321
Flowchart:
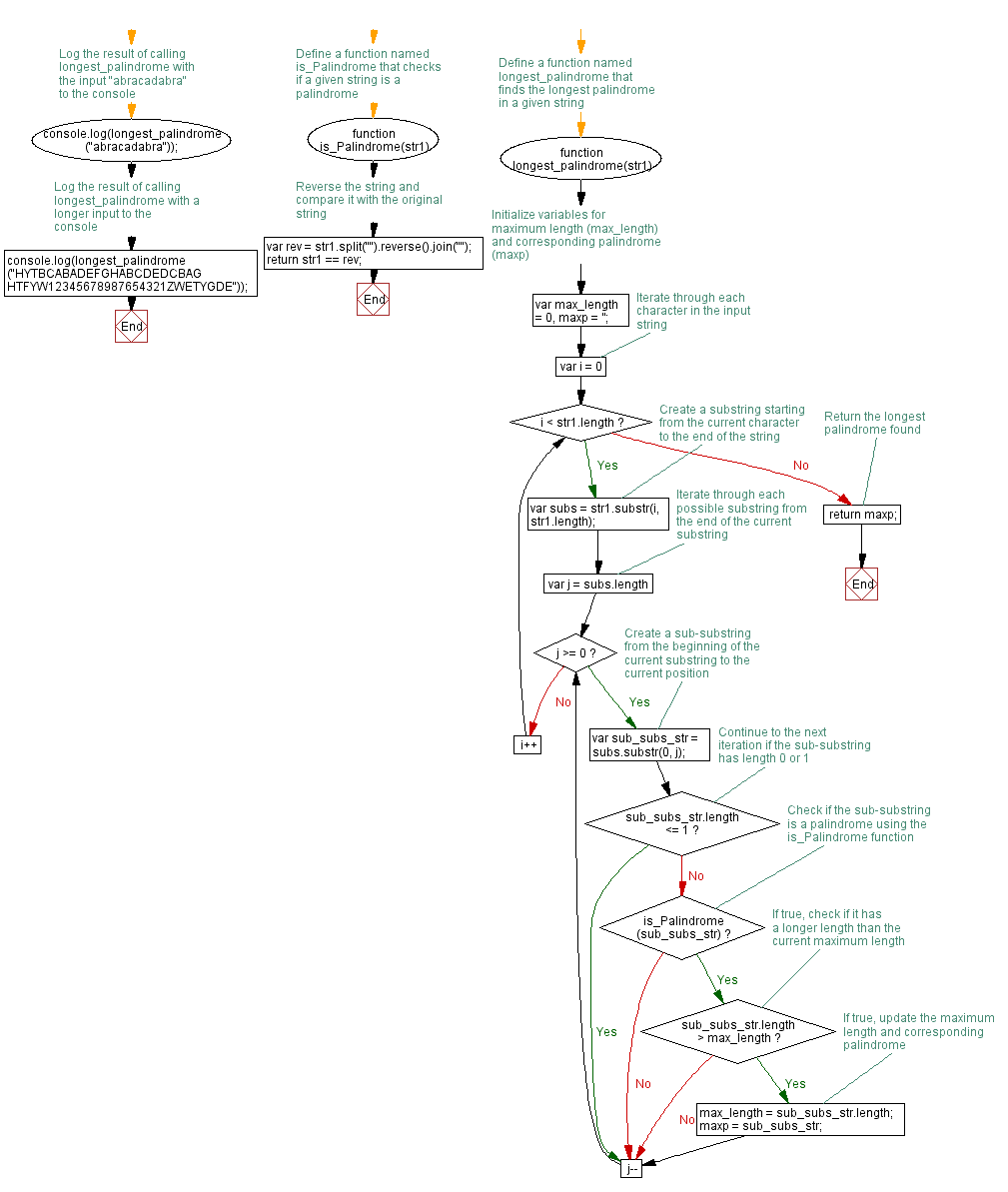
Live Demo:
See the Pen JavaScript - The longest palindrome in a specified string-function-ex- 27 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Helper function to check if a given string is a palindrome
function isPalindrome(str) {
return str === str.split('').reverse().join('');
}
// Function to find the longest palindrome in a given string
function longest_palindrome(str) {
let maxLength = 0; // Variable to track the maximum length of a palindrome
let longestPalindrome = ''; // Variable to store the longest palindrome found
// Nested loops to iterate through all possible substrings in the input string
for (let i = 0; i < str.length; i++) {
for (let j = i + 1; j <= str.length; j++) {
let substring = str.slice(i, j); // Extract the current substring
// Check if the substring is a palindrome and longer than the current maximum
if (isPalindrome(substring) && substring.length > maxLength) {
maxLength = substring.length; // Update the maximum length
longestPalindrome = substring; // Update the longest palindrome
}
}
}
return longestPalindrome; // Return the longest palindrome found
}
// Example usage:
console.log(longest_palindrome("abracadabra")); // Output: "aca"
console.log(longest_palindrome("HYTBCABADEFGHABCDEDCBAGHTFYW12345678987654321ZWETYGDE")); // Output: "12345678987654321"
Output:
aca 12345678987654321
Flowchart:
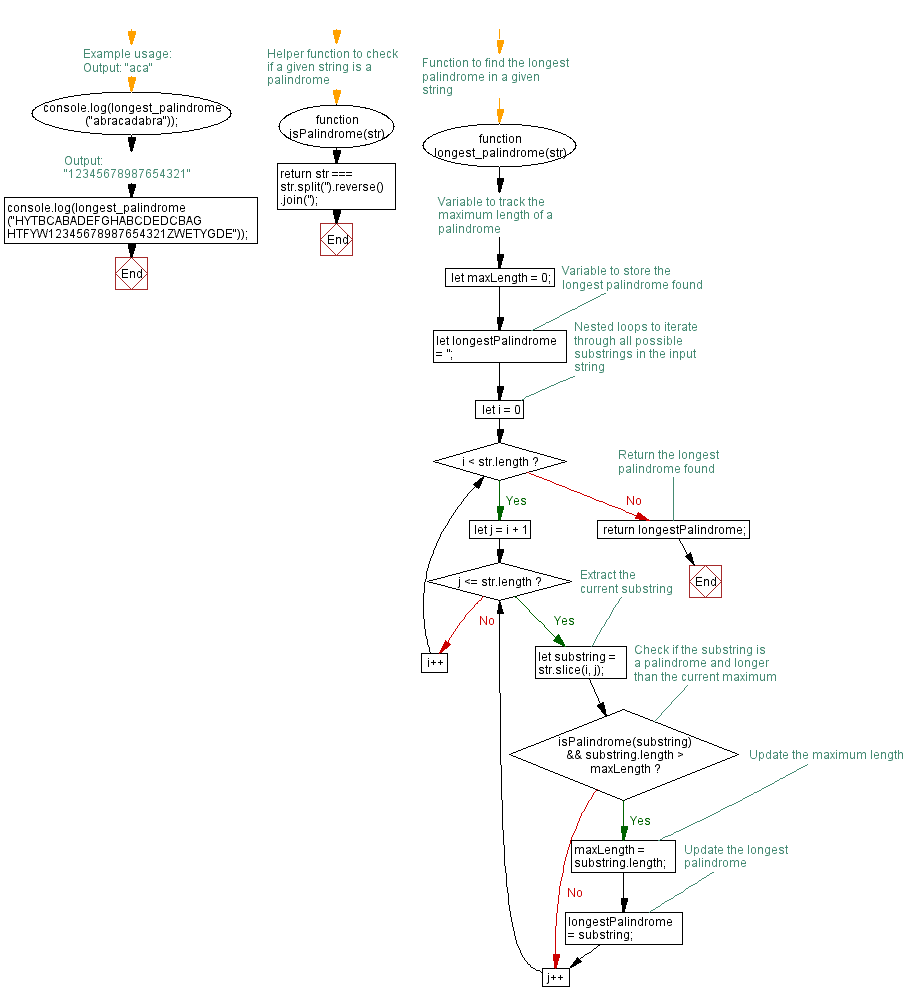
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the longest palindromic substring using dynamic programming.
- Write a JavaScript function that returns the longest palindromic substring by expanding around potential centers.
- Write a JavaScript function that computes the longest palindromic substring in a string using nested loops for comparison.
- Write a JavaScript function that finds all palindromic substrings in a string and returns the longest one among them.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find longest substring in a given a string without repeating characters.
Next: Write a JavaScript program to pass a 'JavaScript function' as parameter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.