JavaScript: Get the data type
JavaScript Function: Exercise-9 with Solution
Get Type of Argument
Write a JavaScript function that accepts an argument and returns the type.
Note : There are six possible values that typeof returns: object, boolean, function, number, string, and undefined.
Sample Solution-1:
JavaScript Code:
// Define a function named detect_data_type that determines the data type of the given value
function detect_data_type(value) {
// Define an array of data types to check against: Function, RegExp, Number, String, Boolean, Object
var dtypes = [Function, RegExp, Number, String, Boolean, Object], x, len;
// Check if the value is an object or a function
if (typeof value === "object" || typeof value === "function") {
// Iterate through the array of data types
for (x = 0, len = dtypes.length; x < len; x++) {
// If the value is an instance of the current data type, return the data type
if (value instanceof dtypes[x]) {
return dtypes[x];
}
}
}
// If the value is not an object or function, return its typeof value
return typeof value;
}
// Log the result of calling detect_data_type with the input value 12 to the console
console.log(detect_data_type(12));
// Log the result of calling detect_data_type with the input value 'w3resource' to the console
console.log(detect_data_type('w3resource'));
// Log the result of calling detect_data_type with the input value false to the console
console.log(detect_data_type(false));
Output:
number string boolean
Flowchart:
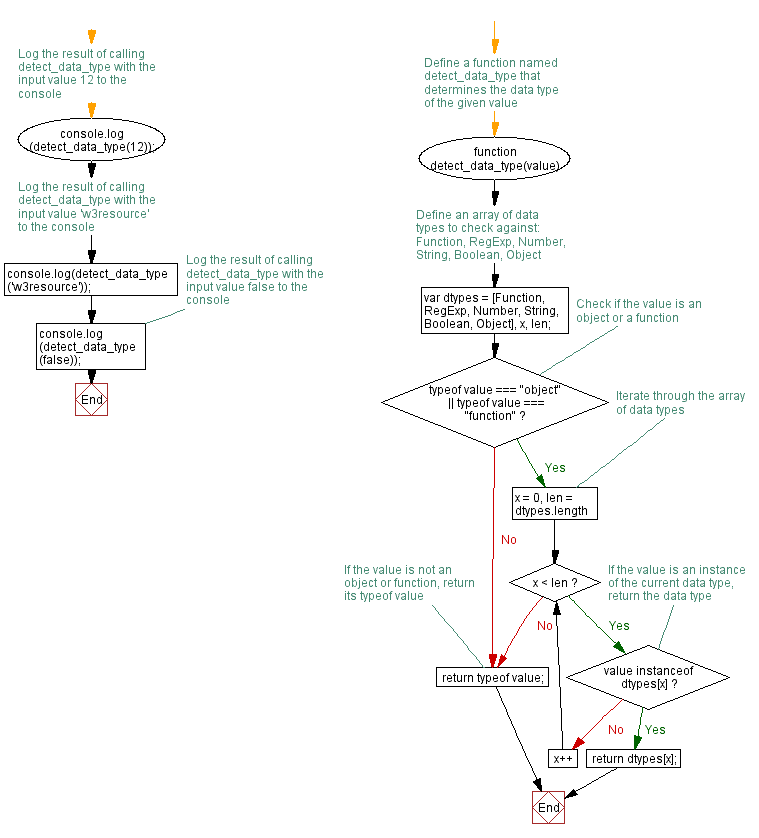
Live Demo:
See the Pen JavaScript -Check a number is prime or not-function-ex- 8 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
function getType(value) {
const typeString = Object.prototype.toString.call(value);
// Extract the type from the string, removing brackets and the "object " prefix
return typeString.slice(8, -1).toLowerCase();
}
// Example usage:
console.log(getType("Hello")); // Output: string
console.log(getType(42)); // Output: number
console.log(getType(true)); // Output: boolean
console.log(getType(null)); // Output: null
console.log(getType(undefined));// Output: undefined
console.log(getType([])); // Output: array
console.log(getType({})); // Output: object
Output:
string number boolean null undefined array object
Flowchart:
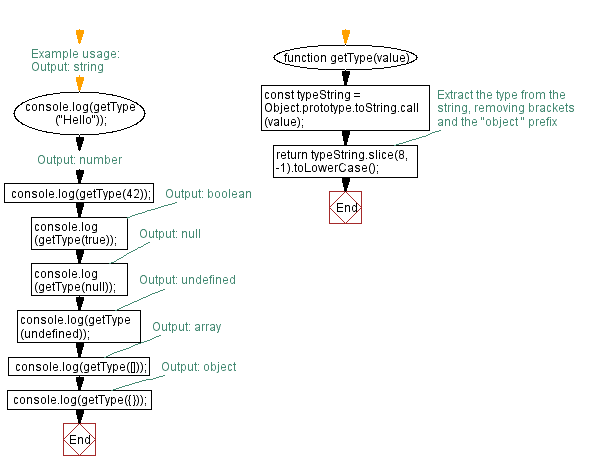
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the type of a given argument and correctly distinguishes between null and object.
- Write a JavaScript function that identifies whether an argument is an array, object, or function using both typeof and instanceof.
- Write a JavaScript function that returns a string indicating the type of a variable, including support for custom class instances.
- Write a JavaScript function that checks an argument’s type and throws an error if it does not match one of the expected types.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that accepts a number as a parameter and check the number is prime or not.
Next: Write a JavaScript function which returns the n rows by n columns identity matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.