JavaScript: Power of four
JavaScript Math: Exercise-108 with Solution
Write a JavaScript program to check whether a given integer is a power of four or not.
In arithmetic and algebra, the fourth power of a number n is the result of multiplying four instances of n together. So: n4 = n × n × n × n
Fourth powers are also formed by multiplying a number by its cube. Furthermore, they are squares of squares.
The sequence of fourth powers of integers (also known as biquadrates or tesseractic numbers) is:
0, 1, 16, 81, 256, 625, 1296, 2401, 4096, 6561, 10000, 14641, 20736, 28561, 38416, 50625, 65536, 83521, 104976, 130321, 160000, 194481, 234256, 279841, 331776, 390625, 456976, 531441, 614656, 707281, 810000, ...
Test Data:
(16) -> true
(4096) -> true
(36) -> false
Sample Solution:
Solution-1
JavaScript Code:
/**
* Function to check if a number is a power of four.
* @param {number} n - The number to be checked.
* @returns {boolean} - True if the number is a power of four, false otherwise.
*/
function test(n) {
// Check if the number is less than 1
if (n < 1) {
return false;
}
// Divide the number by 4 until it is not divisible by 4
while (n % 4 === 0) {
n = n / 4;
}
// Check if the resulting number is 1
return n === 1;
}
// Test cases
n = 16;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
n = 4096;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
n = 36;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
Output:
Number n = 16 Check the said number is power of four or not? true Number n = 4096 Check the said number is power of four or not? true Number n = 36 Check the said number is power of four or not? false
Flowchart:
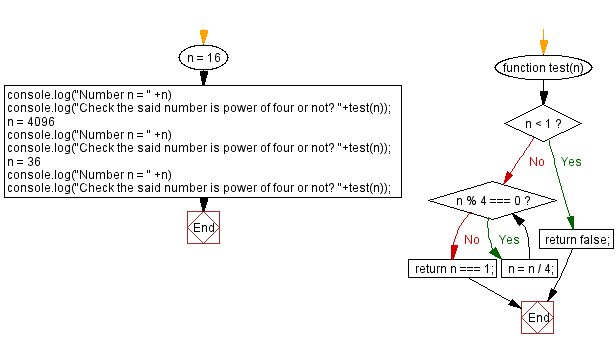
Live Demo:
See the Pen javascript-math-exercise-108 by w3resource (@w3resource) on CodePen.
Solution-2
JavaScript Code:
/**
* Function to check if a number is a power of four.
* @param {number} n - The number to be checked.
* @returns {boolean} - True if the number is a power of four, false otherwise.
*/
function test(n) {
// Check if the number is 1 or 4
if(n === 1 || n === 4) return true;
// Check if the number is less than 4
if(n < 4) return false;
// Recursively call the function with n divided by 4
return test(n / 4);
}
// Test cases
n = 16;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
n = 4096;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
n = 36;
console.log("Number n = " + n);
console.log("Check if the said number is a power of four: " + test(n));
Output:
Number n = 16 Check the said number is power of four or not? true Number n = 4096 Check the said number is power of four or not? true Number n = 36 Check the said number is power of four or not? false
Flowchart:
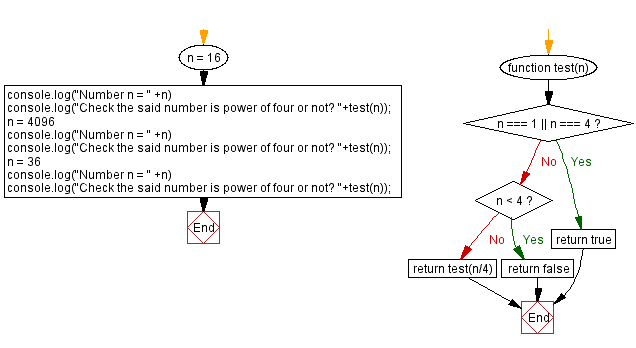
Live Demo:
See the Pen javascript-math-exercise-108-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Power of three.
Next: Count all numbers with unique digits in a range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-math-exercise-108.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics