JavaScript: Sum of two square numbers equal to an integer
JavaScript Math: Exercise-110 with Solution
Sum of Two Squares Equals Integer
Write a JavaScript program to check whether the sum of two square integers is equal to another given integer.
For example a2 + b2 = c where c is the given integer.
Example: 13 = 22 + 32
Test Data:
(2) -> true
(5) -> true
(13) -> true
(15) -> false
Sample Solution:
Solution-1
JavaScript Code:
/**
* Function to check if a given integer can be represented as the sum of two squares.
* @param {number} n - The integer to check.
* @returns {boolean} - True if the integer can be represented as the sum of two squares, otherwise false.
*/
function test(n) {
// If n is less than or equal to 1, it cannot be represented as the sum of two squares
if (n <= 1) return false;
// Calculate the maximum value of the first square (integer part of square root of n - 1)
max_val = Math.floor(Math.sqrt(n - 1));
// Iterate from max_val down to 0
while (max_val >= 0) {
// Calculate the square root of (n - max_val^2)
temp = Math.sqrt(n - max_val * max_val);
// If temp is an integer (no remainder when divided by 1), return true
if (!(temp % 1)) {
return true;
};
// Decrement max_val for the next iteration
max_val -= 1;
};
// If no valid combination is found, return false
return false;
}
// Test cases
n = 2;
console.log("n = " + n);
console.log("Check if the sum of two square integers is equal to the said integer: " + test(n));
n = 5;
console.log("n = " + n);
console.log("Check if the sum of two square integers is equal to the said integer: " + test(n));
n = 13;
console.log("n = " + n);
console.log("Check if the sum of two square integers is equal to the said integer: " + test(n));
n = 15;
console.log("n = " + n);
console.log("Check if the sum of two square integers is equal to the said integer: " + test(n));
Output:
n = 2 Check sum of two square integers is equal to said integer: true n = 5 Check sum of two square integers is equal to said integer: true n = 13 Check sum of two square integers is equal to said integer: true n = 15 Check sum of two square integers is equal to said integer: false
Flowchart:
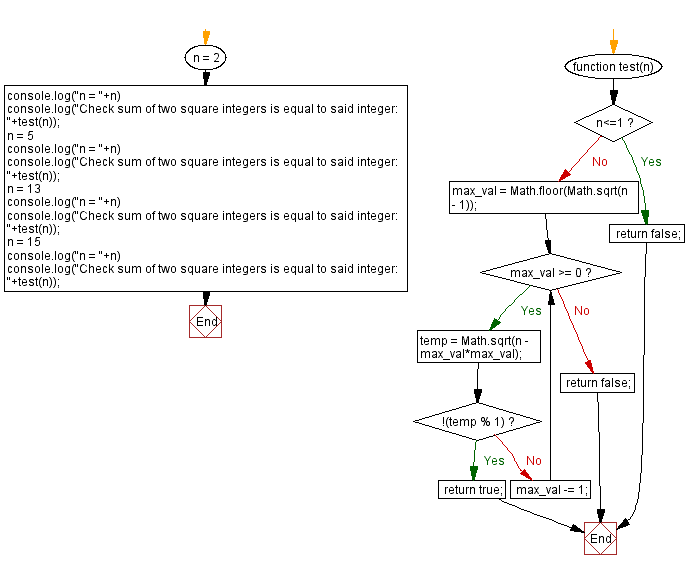
Live Demo:
See the Pen javascript-math-exercise-110 by w3resource (@w3resource) on CodePen.
Solution-2
JavaScript Code:
/**
* Function to check if a given integer can be represented as the sum of two square integers.
* @param {number} n - The integer to check.
* @returns {boolean} - True if the integer can be represented as the sum of two square integers, otherwise false.
*/
function test(n) {
// If n is less than or equal to 1, it cannot be represented as the sum of two square integers
if (n <= 1) return false;
// Iterate over i and j from 0 to the square root of n
for(var i = 0; i * i <= n; i++){
for(var j = 0; j * j <= n; j++) {
// Calculate the squares of i and j
var a = i * i;
var b = j * j;
// If the sum of squares is equal to n, return true
if (a + b == n)
return true;
}
}
// If no valid combination is found, return false
return false;
}
// Test cases
n = 2;
console.log("n = " + n);
console.log("Check if the sum of two square integers is equal to the said integer: " + test(n));
n = 5;
console.log("n = " + n);
console.log("Check if the sum of two square integers is equal to the said integer: " + test(n));
n = 13;
console.log("n = " + n);
console.log("Check if the sum of two square integers is equal to the said integer: " + test(n));
n = 15;
console.log("n = " + n);
console.log("Check if the sum of two square integers is equal to the said integer: " + test(n));
Output:
n = 2 Check sum of two square integers is equal to said integer: true n = 5 Check sum of two square integers is equal to said integer: true n = 13 Check sum of two square integers is equal to said integer: true n = 15 Check sum of two square integers is equal to said integer: false
Flowchart:
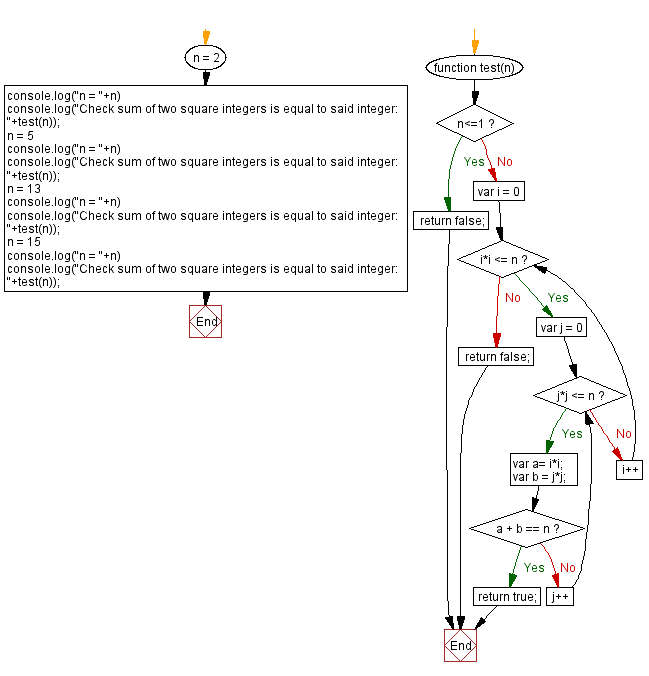
Live Demo:
See the Pen javascript-math-exercise-110-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a given integer can be represented as the sum of two square numbers.
- Write a JavaScript function that iterates through possible square numbers and tests if any two add up to the given integer.
- Write a JavaScript function that uses nested loops to examine combinations of squares up to the square root of the integer.
- Write a JavaScript function that validates the input and returns a boolean indicating if the sum of two squares equals the integer.
Go to:
PREV : Unique Digit Numbers Count.
NEXT : Kth Smallest in Multiplication Table.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.