JavaScript: Check a given number is self-dividing or not
JavaScript Math: Exercise-114 with Solution
Check Self-Dividing Number
Write a JavaScript program that checks if a given number is self-dividing.
In mathematics, a self-dividing number is one that can be divided by every digit (except digit zero) within it.
Test Data:
(48) -> true
(22) -> true
(63) -> false
Sample Solution:
JavaScript Code:
/**
* Function to check if a number is self-dividing or not.
* @param {number} n - The number to be checked.
* @returns {boolean} - True if the number is self-dividing, false otherwise.
*/
function test(n) {
i = n; // Initialize i to n
num = i; // Store the value of n in num
flag = true; // Initialize flag to true
while (num > 0) { // Loop through each digit of the number
mod = num % 10; // Get the last digit of num
if (i % mod !== 0) { // Check if i is divisible by mod
flag = false; // If not, set flag to false
}
num = Math.floor(num / 10); // Remove the last digit from num
}
return flag; // Return the value of flag indicating if the number is self-dividing or not
}
// Test cases
n = 48;
console.log("n = " + n);
console.log("Check the said number is self-dividing or not: " + test(n));
n = 22;
console.log("n = " + n);
console.log("Check the said number is self-dividing or not: " + test(n));
n = 63;
console.log("n = " + n);
console.log("Check the said number is self-dividing or not: " + test(n));
Output:
n = 48 Check the said number is self-dividing or not: true n = 22 Check the said number is self-dividing or not: true n = 63 Check the said number is self-dividing or not: false
Flowchart:
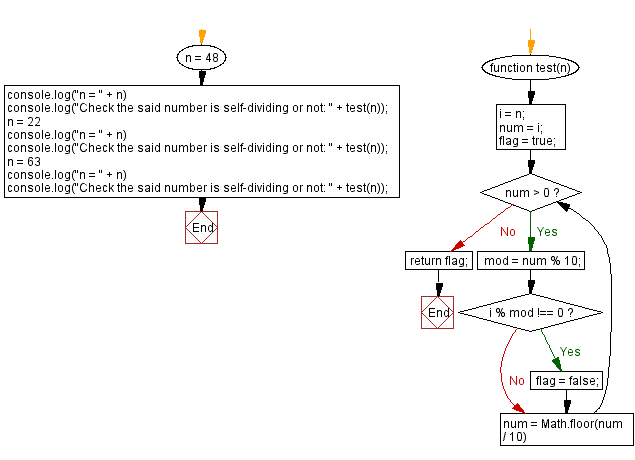
Live Demo:
See the Pen javascript-math-exercise-114 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if every non-zero digit of a number divides the number evenly.
- Write a JavaScript function that converts a number to a string and verifies that each digit (ignoring zero) is a divisor of the number.
- Write a JavaScript function that iterates through the digits of a number and returns true if all non-zero digits are factors.
- Write a JavaScript function that validates input and handles special cases, such as numbers containing zeros, appropriately.
Go to:
PREV : Smallest Number from Digit Product.
NEXT : Javascript Array Exercises Home
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.