JavaScript: Find out if a number is a natural number or not
JavaScript Math: Exercise-12 with Solution
Write a JavaScript function to find out if a number is a natural number or not.
Note:
Natural numbers are whole numbers from 1 upwards : 1, 2, 3, and so on ...
or from 0 upwards in some area of mathematics: 0, 1, 2, 3 and so on ...
No negative numbers and no fractions.
Test Data:
console.log(is_Natural(-15));
console.log(is_Natural(1));
console.log(is_Natural(10.22));
console.log(is_Natural(10/0));
Output:
false
true
false
false
Visual Presentation:
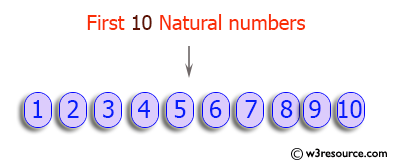
Sample Solution:
JavaScript Code:
// Define a function named is_Natural that checks if a number is a natural number.
function is_Natural(n)
{
// Check if the input is not a number, if so, return 'Not a number'.
if (typeof n !== 'number')
return 'Not a number';
// Check if the number is non-negative, an integer, finite, and not Infinity.
return (n >= 0.0) && (Math.floor(n) === n) && n !== Infinity;
}
// Output the result of checking if -15 is a natural number to the console.
console.log(is_Natural(-15));
// Output the result of checking if 1 is a natural number to the console.
console.log(is_Natural(1));
// Output the result of checking if 10.22 is a natural number to the console.
console.log(is_Natural(10.22));
// Output the result of checking if 10/0 is a natural number to the console.
console.log(is_Natural(10/0));
Output:
false true false false
Flowchart:
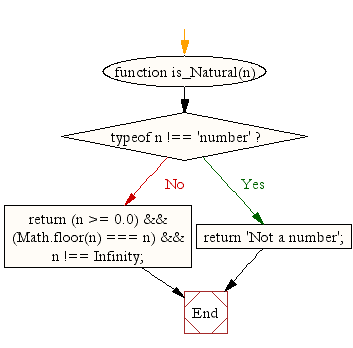
Live Demo:
See the Pen javascript-math-exercise-12 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get the least common multiple (LCM) of more than 2 integers.
Next: Write a JavaScript function to test if a number is a power of 2.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics