JavaScript: Convert Roman Numeral to Integer
JavaScript Math: Exercise-22 with Solution
Roman Numeral to Integer Conversion
Write a JavaScript function that converts Roman numerals to integers.
Sample Solution:
JavaScript Code:
// Define a function named roman_to_Int that converts a Roman numeral to an integer.
function roman_to_Int(str1) {
// Check if str1 is null, if so, return -1.
if(str1 == null) return -1;
// Initialize the variable num with the integer value of the first character in str1.
var num = char_to_int(str1.charAt(0));
var pre, curr;
// Iterate through the characters of str1 starting from the second character.
for(var i = 1; i < str1.length; i++){
curr = char_to_int(str1.charAt(i)); // Get the integer value of the current character.
pre = char_to_int(str1.charAt(i-1)); // Get the integer value of the previous character.
// If the current character's value is less than or equal to the previous character's value, add it to num.
if(curr <= pre){
num += curr;
} else {
// If the current character's value is greater than the previous character's value, subtract twice the previous character's value and add the current character's value to num.
num = num - pre*2 + curr;
}
}
// Return the final integer value of the Roman numeral.
return num;
}
// Define a function named char_to_int that returns the integer value of a Roman numeral character.
function char_to_int(c){
switch (c){
case 'I': return 1;
case 'V': return 5;
case 'X': return 10;
case 'L': return 50;
case 'C': return 100;
case 'D': return 500;
case 'M': return 1000;
default: return -1; // Return -1 for invalid characters.
}
}
// Output the integer value of the Roman numeral 'XXVI' to the console.
console.log(roman_to_Int('XXVI'));
// Output the integer value of the Roman numeral 'CI' to the console.
console.log(roman_to_Int('CI'));
Output:
26 101
Visual Presentation:
Flowchart:
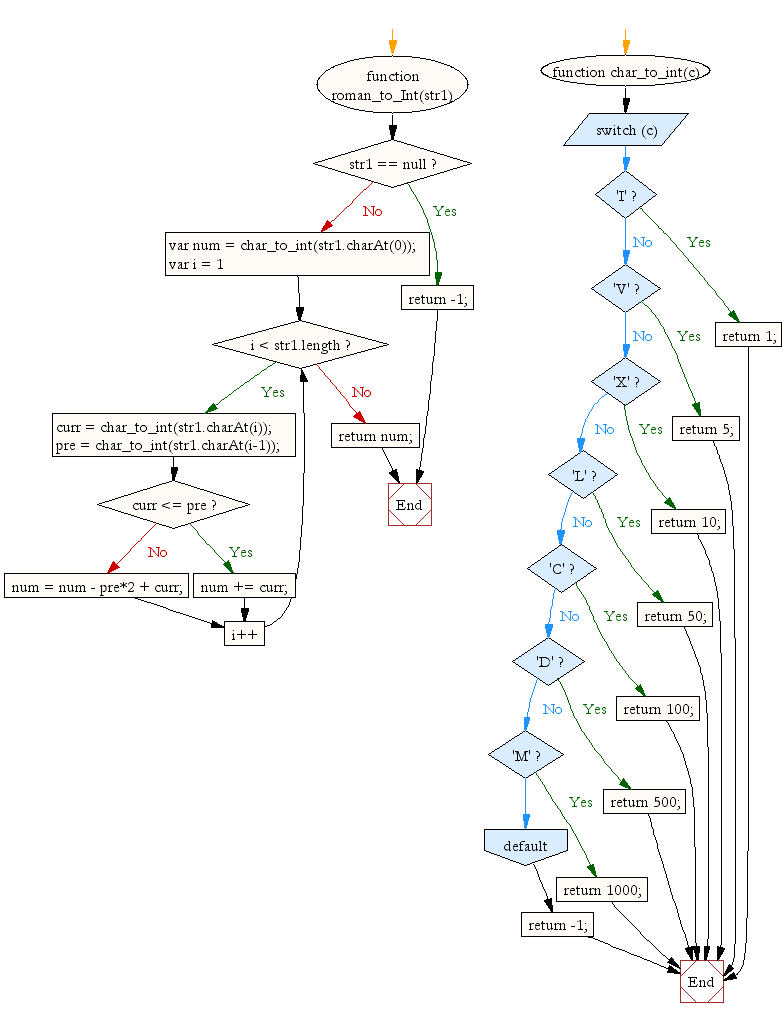
Live Demo:
See the Pen javascript-math-exercise-22 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that converts a Roman numeral string to its integer value by mapping symbols to numbers.
- Write a JavaScript function that parses a Roman numeral using subtraction rules and computes the corresponding integer.
- Write a JavaScript function that validates a Roman numeral string and converts it to an integer, handling errors for invalid input.
- Write a JavaScript function that iterates through a Roman numeral and adds or subtracts values based on the order of symbols.
Go to:
PREV : Integer to Roman Numeral Conversion.
NEXT : Generate UUID Identifier.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.