JavaScript: Cast a square root of a number to an integer
JavaScript Math: Exercise-30 with Solution
Write a JavaScript function to cast the square root of a number to an integer.
Test Data:
console.log(sqrt_to_int(17));
4
Sample Solution:
JavaScript Code:
// Define a function named sqrt_to_int that calculates the square root of a number and returns the integer part of the result.
function sqrt_to_int(num)
{
// Compute the square root of num using Math.sqrt(), convert it to a string, and parse it to an integer using parseInt().
return parseInt(Math.sqrt(num)+"");
}
// Output the result of calculating the square root of 17 and converting it to an integer to the console.
console.log(sqrt_to_int(17));
Output:
4
Flowchart:
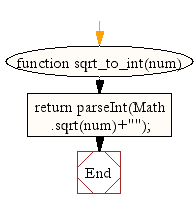
Live Demo:
See the Pen javascript-math-exercise-30 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert a positive number to negative number.
Next: Write a JavaScript function to get the highest number from three different numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-math-exercise-30.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics